Image Carousel is a Carousel that allows images to be displayed as it revolves around, in this case it is a Vertical Carousel using Silverlight on Windows Phone 7.
Printer Friendly Download Tutorial (905KB) Download Source Code (19.9KB)
Step 1
Start Microsoft Visual Studio 2010 Express for Windows Phone, then Select File then New Project... Select "Visual C#" then "Silverlight for Windows Phone" and then "Windows Phone Application" from Templates, select a Location if you wish, then enter a name for the Project and then click OK, see below:
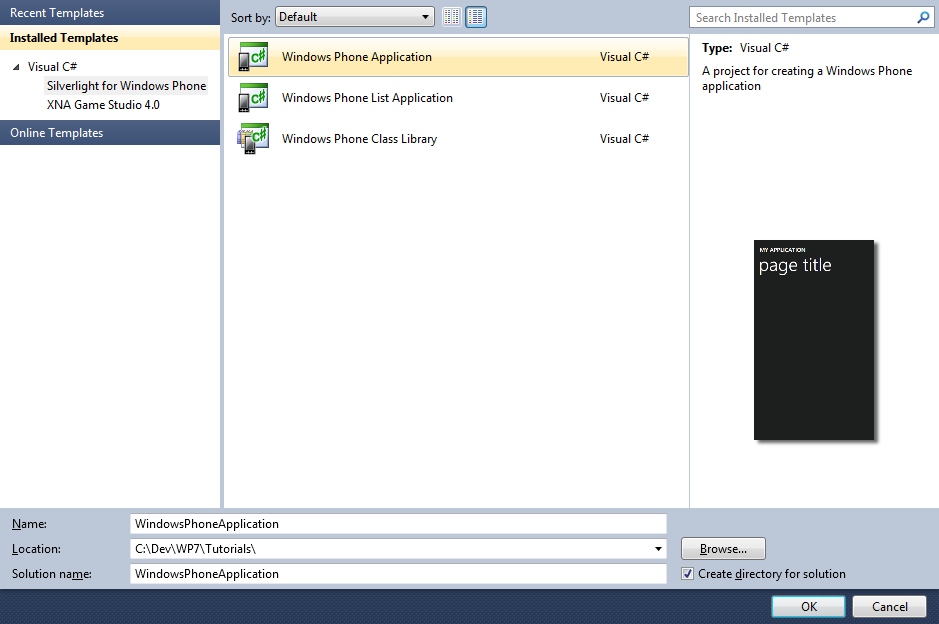
Step 2
A Windows Phone application Page named MainPage.xaml should then appear, see below:
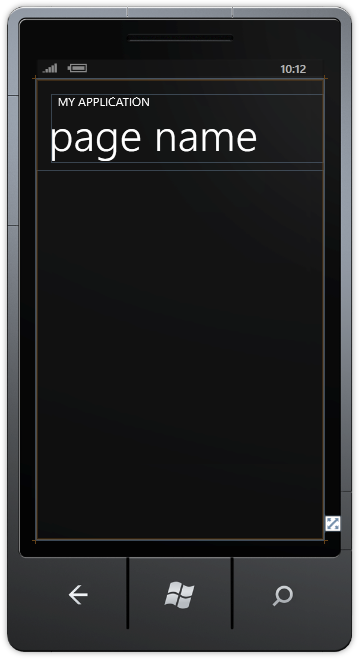
Step 3
Right Click on the Entry for the Project in the Solution Explorer and choose "Add" then "New Folder", and give it the Name "images" (without quotes), see below:
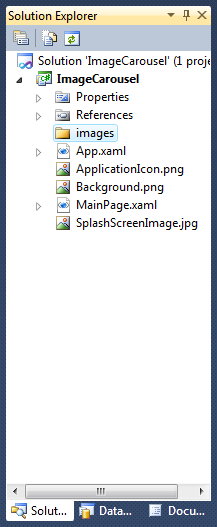
Step 4
Download the following images (add.png, remove.png & clear.png) by right-clicking on the images below and choose "Save Picture As..." or "Save Image As..." and Save them to a Folder on your computer:
Step 5
Right Click on the Entry for the "image" Folder for the Project in Solution Explorer, and choose "Add", then "Existing Item...", then in the "Add Existing Item" dialog select Folder you saved the images, then choose "Add" to add add.png, remove.png and clear.png to the images folder in the project, see below:
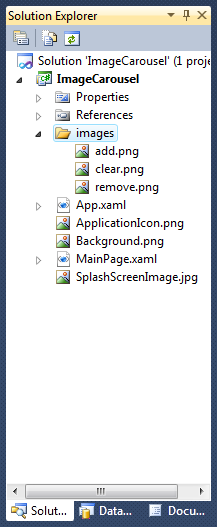
Step 6
While still in the Solution Explorer click on the "add.png" image then goto the Properties box and change the Build Action to Content, do the same for the "clear.png" and "remove.png" images, see below:
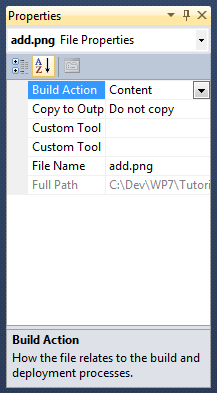
Step 7
Select the ImageCarousel Project from Solution Explorer.
Then select from the Main Menu "Project" then "Add New Item...", and select the "Windows Phone User Control" Template, then change the "Name" to CarouselItem.xaml see below:
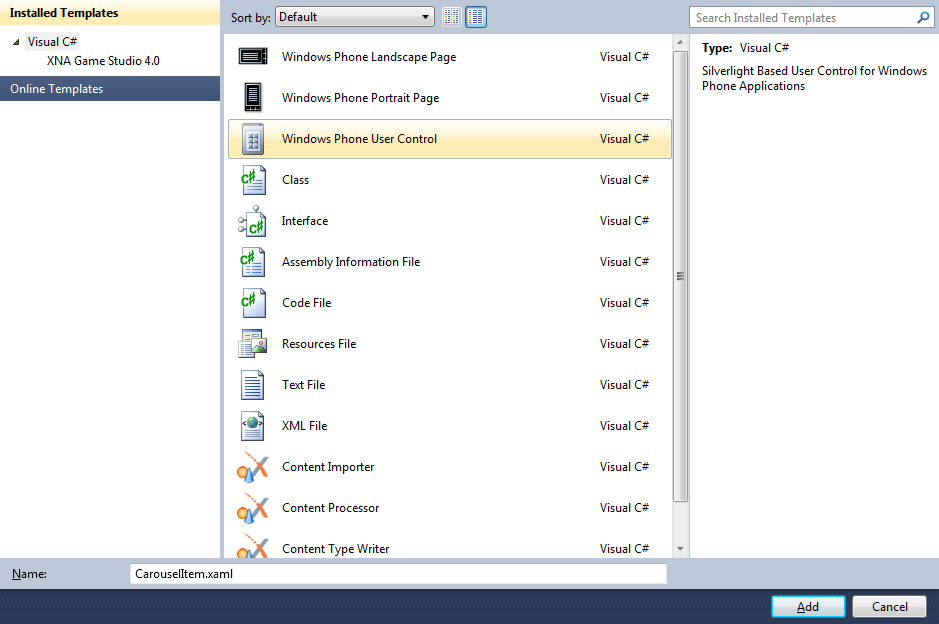
Step 8
Add the new User Control to the Project by Clicking on Add, then in the XAML Pane for the CarouselItem.xaml User Control.
Remove d:DesignHeight="480" d:DesignWidth="480"
from the <UserControl ...> Tag.
Also remove Background="{StaticResource PhoneChromeBrush}" from <Grid x:Name="LayoutRoot" ...>, see below:
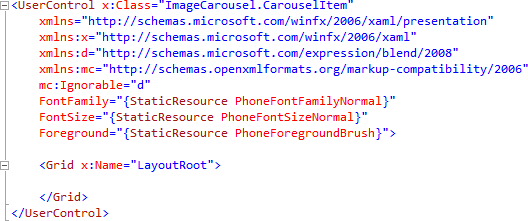
Step 9
While still in XAML Pane for CarouselItem.xaml between the <Grid x:Name="LayoutRoot"> and </Grid> lines type the following Image Control XAML:
<Image Height="150" Width="150" Name="Image">
<Image.RenderTransform>
<TransformGroup>
<ScaleTransform ScaleX="1" ScaleY="1" x:Name="ItemScale"/>
</TransformGroup>
</Image.RenderTransform>
</Image>
XAML:
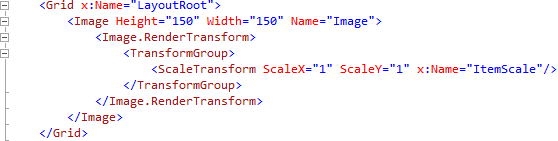
Design:
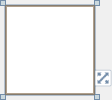
Step 10
Right Click on the Page or the entry for "CarouselItem.xaml" in Solution Explorer and choose the "View Code" option. In the Code View above "public CarouselItem()" type the following Declaration:
public double Angle = 0;
See Below:
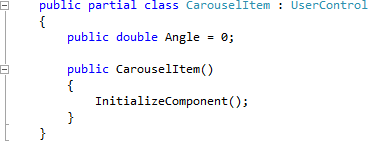
Step 11
Select from the Main Menu "Project" then "Add New Item...", and select the "Windows Phone User Control" Template, then change the "Name" to Carousel.xaml see below:
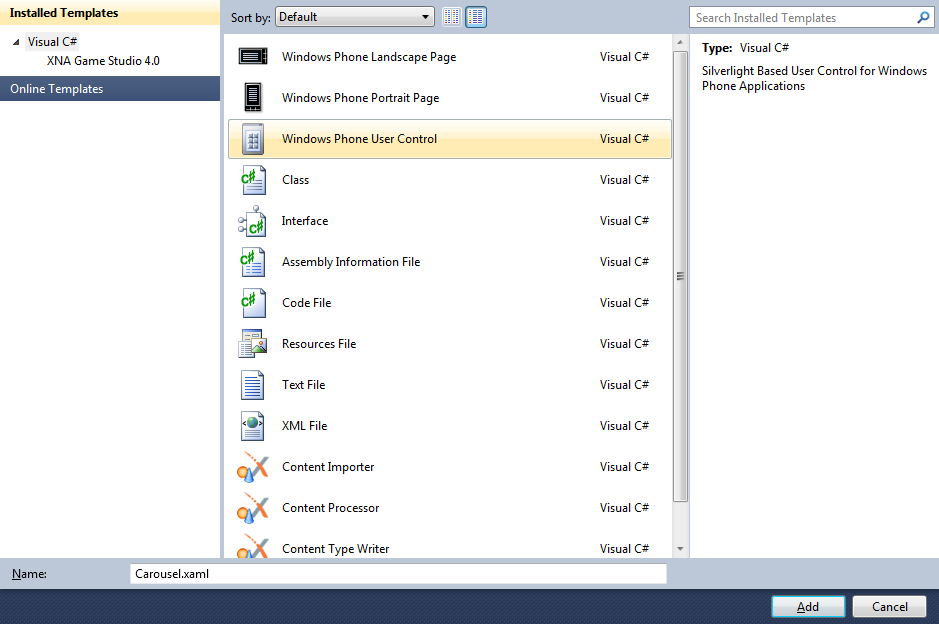
Step 12
Add the new User Control to the Project by Clicking on Add, then in the XAML Pane for the Carousel.xaml User Control.
Remove Background="{StaticResource PhoneChromeBrush}" from <Grid x:Name="LayoutRoot" ...>
from the <UserControl ...> Tag, see below:
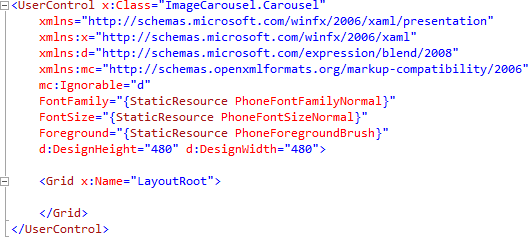
Step 13
While still in XAML Pane for Carousel.xaml between the <Grid x:Name="LayoutRoot"> and </Grid> lines type the following XAML:
<Canvas x:Name="Container" Width="480" Height="480">
<Canvas Height="150" Width="150" x:Name="Display"/>
</Canvas>
XAML:

Design:
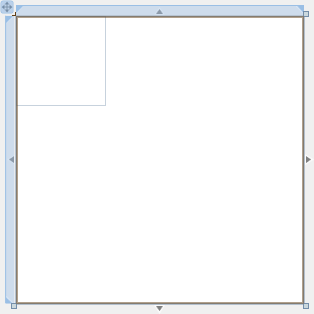
Step 14
Right Click on the Entry for the "Carousel.xaml" Class in Solution Explorer and choose "View Code".
In the Code View for Carousel.xaml.cs above "namespace ImageCarousel", type the following:
using System.Windows.Media.Imaging;
Also in the CodeView below the "{" of the line "public partial class Carousel : UserControl" type the following Declarations:
private Storyboard _rotate = new Storyboard(); private List<BitmapImage> _images = new List<BitmapImage>(); private List<CarouselItem> _items = new List<CarouselItem>(); private Point _position; private Point _radius = new Point { X = -25, Y = 200 }; private double _speed = 0.0125; private double _perspective = 75; private double _distance;
See Below:
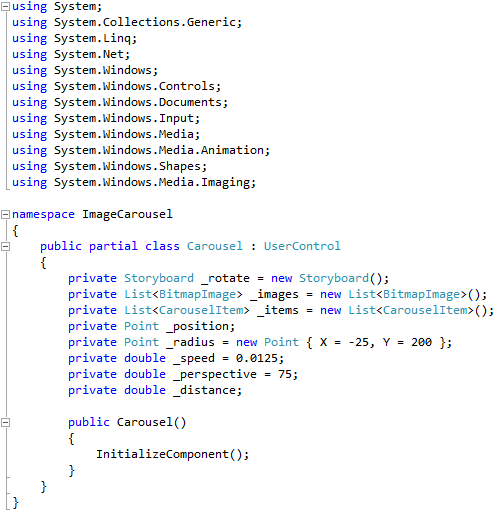
Step 15
While still in the Code View below the "_distance" declaration type the following Methods:
private void Populate(ref Canvas canvas) { canvas.Children.Clear(); for (int index = 0; index < _images.Count(); index++) { CarouselItem item = new CarouselItem(); item.Image.Source = _images[index]; item.Angle = index * ((Math.PI * 2) / _images.Count); _position.X = Math.Cos(item.Angle) * _radius.X; _position.Y = Math.Sin(item.Angle) * _radius.Y; Canvas.SetLeft(item, _position.X); Canvas.SetTop(item, _position.Y); _distance = 1 / (1 - (_position.X / _perspective)); item.Opacity = item.ItemScale.ScaleX = item.ItemScale.ScaleY = _distance; _items.Add(item); canvas.Children.Add(item); } } private void Rotate(object sender, EventArgs e) { foreach (CarouselItem item in _items) { item.Angle -= _speed; _position.X = Math.Cos(item.Angle) * _radius.X; _position.Y = Math.Sin(item.Angle) * _radius.Y; Canvas.SetLeft(item, _position.X); Canvas.SetTop(item, _position.Y); if (_radius.X >= 0) { _distance = 1 * (1 - (_position.X / _perspective)); Canvas.SetZIndex(item, -(int)(_position.X)); } else { _distance = 1 / (1 - (_position.X / _perspective)); Canvas.SetZIndex(item, (int)(_position.X)); } item.Opacity = item.ItemScale.ScaleX = item.ItemScale.ScaleY = _distance; } _rotate.Begin(); } public void Add(Uri uri) { BitmapImage _image = new BitmapImage(uri); _images.Add(_image); Populate(ref Display); } public void RemoveLast() { if (_images.Count > 0) { _images.RemoveAt(_images.Count - 1); Populate(ref Display); } } public void Clear() { _images.Clear(); Populate(ref Display); }
See Below:
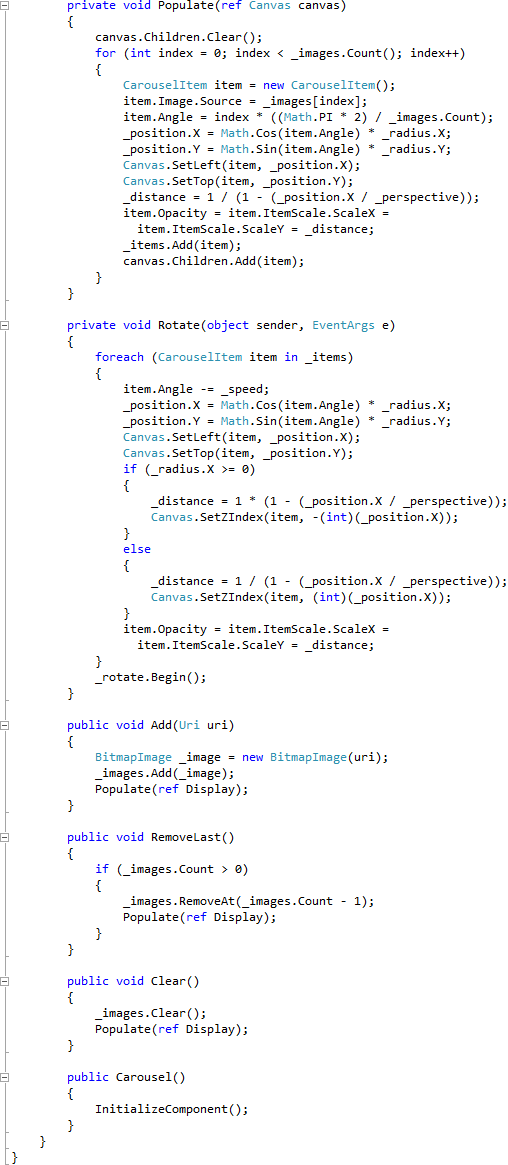
Step 16
While still in the Code View in the "public Carousel()" Constructor below "InitializeComponent();" type the following:
Canvas.SetLeft(Display, Container.Width / 2 - Display.Width / 2); Canvas.SetTop(Display, Container.Height / 2 - Display.Height / 2); _rotate.Completed += Rotate; _rotate.Begin();
See Below:
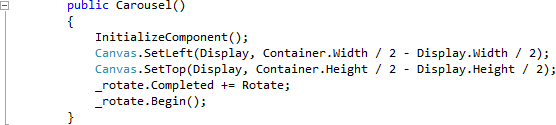
Step 17
Select "Build Solution" from the Debug menu, see below:

Step 18
When the Build completes, return to the MainPage Designer View by selecting the "MainPage.xaml" Tab.
In the XAML Pane for MainPage.xaml, above the <Grid x:Name="LayoutRoot" Background="{StaticResource PhoneBackgroundBrush}"> Tag type the following ApplicationBar XAML:
<phone:PhoneApplicationPage.ApplicationBar>
<shell:ApplicationBar IsVisible="True" IsMenuEnabled="False">
<shell:ApplicationBar.Buttons>
<shell:ApplicationBarIconButton Text="add" IconUri="/images/add.png" Click="Add_Click"/>
<shell:ApplicationBarIconButton Text="remove" IconUri="/images/remove.png" Click="Remove_Click"/>
<shell:ApplicationBarIconButton Text="clear" IconUri="/images/clear.png" Click="Clear_Click"/>
</shell:ApplicationBar.Buttons>
</shell:ApplicationBar>
</phone:PhoneApplicationPage.ApplicationBar>
See below:

Step 19
While still in XAML Pane between the <Grid x:Name="ContentGrid" Grid.Row="1"> and </Grid> lines type the following XAML:
<Grid x:Name="ContentMain">
<Grid.RowDefinitions>
<RowDefinition Height="80"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<!-- Toolbar -->
<!-- Content -->
</Grid>
XAML:
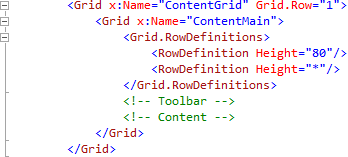
Design:
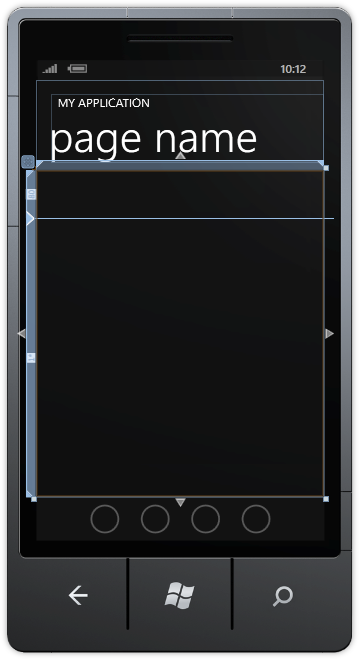
Step 20
Then from the Windows Phone Controls section in the Toolbox select the TextBox control:
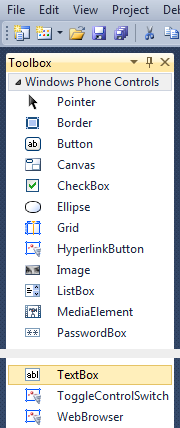
Step 21
Draw a TextBox onto the Toolbar Section (upper smaller section) of the Grid on the Page, below the Page Title, and in the XAML Pane below the <!-- Toolbar --> line, change "TextBox1" to the following:
<TextBox Grid.Row="0" Name="Location">
<TextBox.InputScope>
<InputScope>
<InputScopeName NameValue="Url"/>
</InputScope>
</TextBox.InputScope>
</TextBox>
See below:
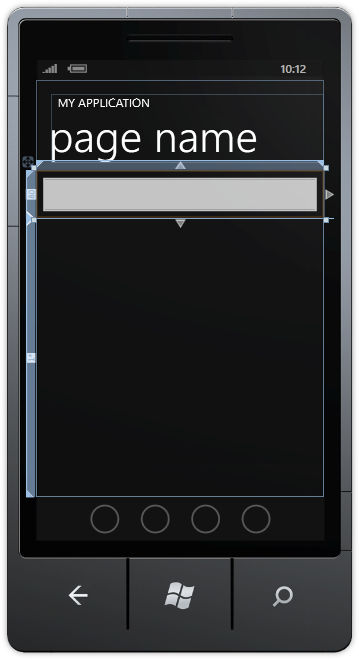
Step 22
Then from the ImageCarousel Controls section in the Toolbox select the Carousel control:
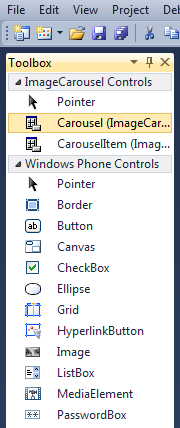
Step 23
Draw a Carousel onto the Page by dragging a Carousel from the Toolbox onto the larger portion of the Grid on the Page (Content), then in the XAML Pane below the <!-- Content --> line, change "carousel1" to the following:
<my:Carousel Grid.Row="1" x:Name="Images"/>
XAML:

Design:
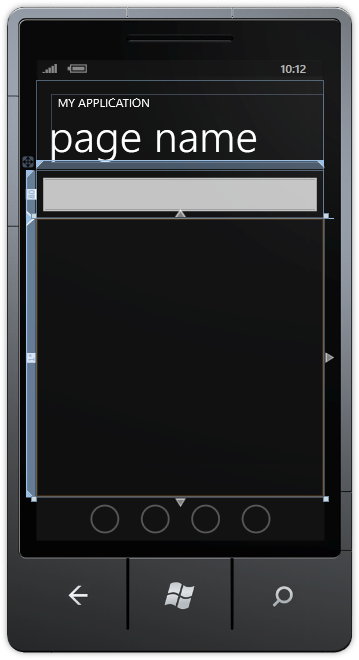
Step 24
While still in the Designer View for MainPage.xaml, Select the "page name" TextBlock (PageTitle) and then Delete or Right-Click and Choose the Delete option so the MainPage.xaml appears as below:
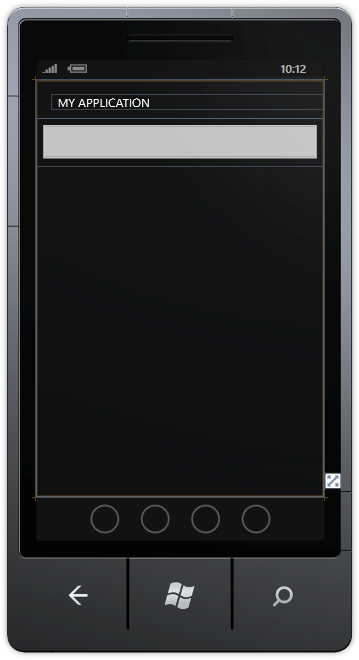
Step 25
Right Click on the Page or the entry for "MainPage.xaml" in Solution Explorer and choose the "View Code" option.
In the Code View in the "public MainPage()" Constructor below "InitializeComponent();" type the following:
ApplicationTitle.Text = "IMAGE CAROUSEL"; Location.Text = "http://cespage.com/phone.jpg";
See Below:
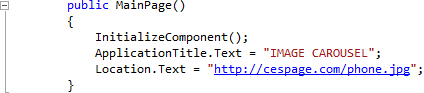
Step 26
While still the Code View for MainPage.xaml.cs above "public MainPage()" type the following Event Handlers:
private void Add_Click(object sender, EventArgs e) { Images.Add(new Uri(Location.Text)); } private void Remove_Click(object sender, EventArgs e) { Images.RemoveLast(); } private void Clear_Click(object sender, EventArgs e) { Images.Clear(); }
See Below:
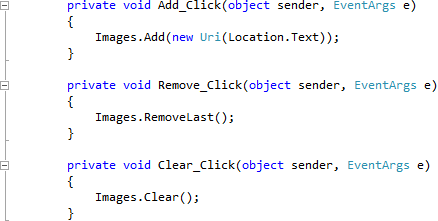
Step 27
Save the Project as you have now finished the Windows Phone Silverlight application. Select the Windows Phone Emulator option then Select Debug then Start Debugging or click on Start Debugging:

After you do, the following will appear in the Windows Phone Emulator after it has been loaded:
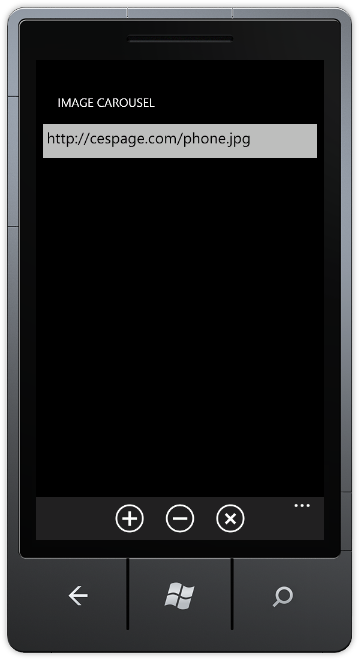
Step 28
Tap the "Location" TextBox and enter using the SIP to enter the URL of an Image then tap the "add" button to Add it to the Carousel, you can do this multiple times to add more Images to the Carousel, see below:
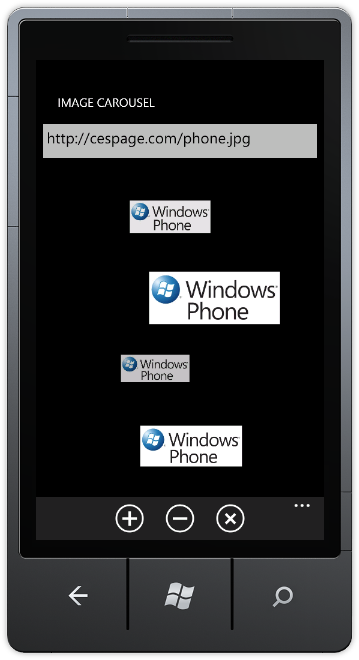
Step 29
You can then Stop the application by selecting the Visual Studio 2010 application window and clicking on the Stop Debugging button:

This is a Simple Image Carousel for displaying images, it could be altered to display any content in a Carousel such as Buttons or other content, try changing it such as making it into a horizontal carousel - make it your own!