Browsing the Internet, is what you are doing now if you're reading this tutorial, which will allow you to implement your very own Web Browser! This uses the Webbrowser Control with Open/Save support for your Favourite websites!
Printer Friendly Download Tutorial (371KB) Download Source Code (13.2KB)
Step 1
Start Microsoft Visual Basic 2008 Express Edition, then select File then New Project... Choose Windows Forms Application from the New Project Window, enter a name for the Project and then click OK, see below:
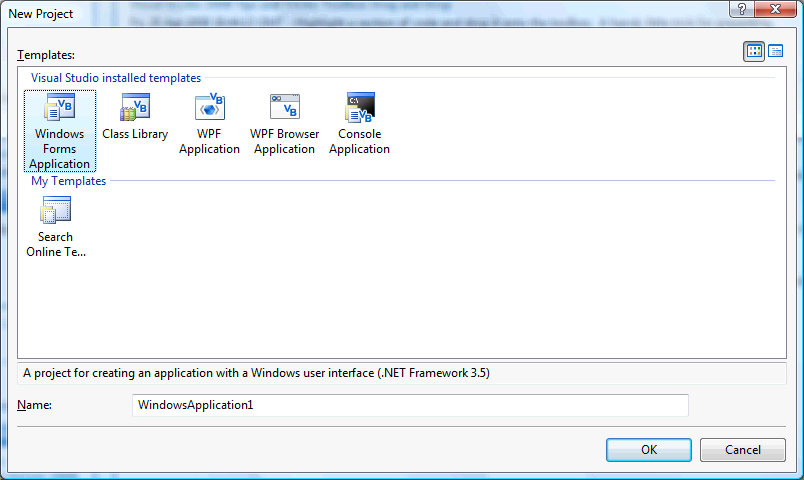
Step 2
A Blank Form named Form1 should then appear, see below:
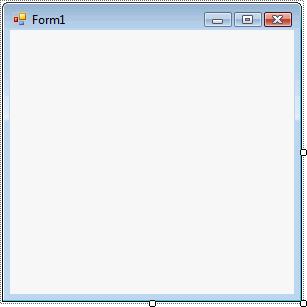
Step 3
With Form1 selected goto the Properties box and change the Name from Form1 to frmMain
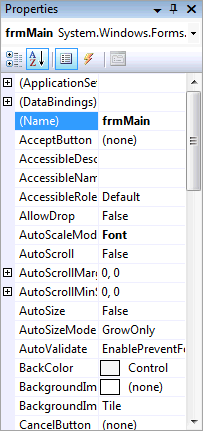
Step 4
Then from the Common Controls tab on the Toolbox select the WebBrowser component:
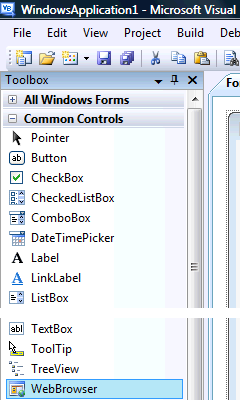
Step 5
Draw a WebBrowser on the Form, make sure to position and size the WebBrowser so it appears as below:
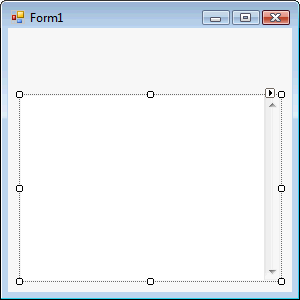
Step 6
Then from the Common Controls tab on the Toolbox select the Textbox component:
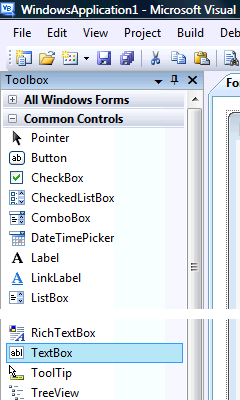
Step 7
Draw a Textbox on the Form, this should be just above the WebBrowser, see below:
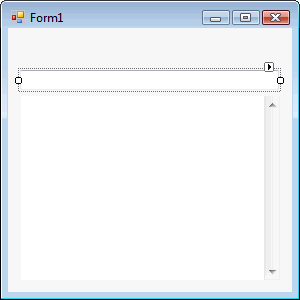
Step 8
Select or click on the Textbox if it is not already selected, then goto the Properties box and change the name to "txtURL", then set the Anchor property to "Top, Left, Right" and set the TabIndex Property to "0", all without quotes, see below:
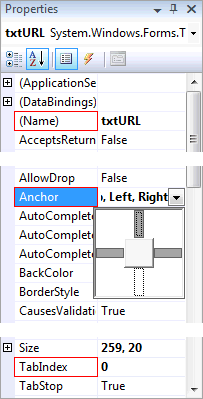
Step 9
Select or click on the WebBrowser, then goto the Properties box and change the name to "webMain", then set the Anchor property to "Top, Bottom, Left, Right" and set the TabIndex Property to "1", all without quotes, see below:
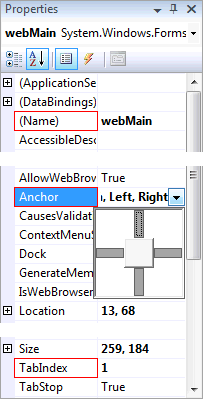
Step 10
Then from the Menus & Toolbars tab on the Toolbox select the MenuStrip Control, see Below:
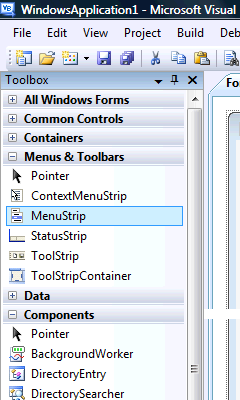
Step 11
Either double-click on the MenuStrip Control entry in the Menu & Toolbars tab on the Toolbox or keep the MenuStrip Component Clicked and then move it over the Form then let go. Change the Name of the MenuStrip in the properties to mnuWeb The Form will then look as below:
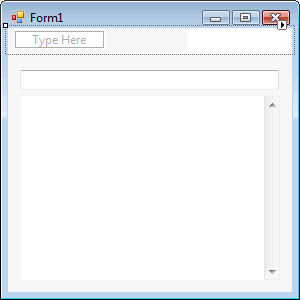
Step 12
Click or select where the MenuStrip says "Type Here", an editable Textbox will appear type in "File" without the quotes in this Box, then in the Box below that type in "Open...", then below that "Save..." and finally "Exit". The MenuStrip should look as below:
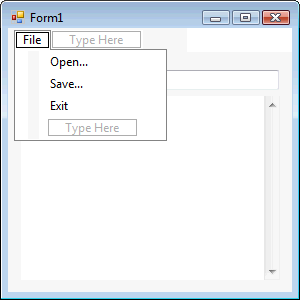
Step 13
Click or select next to "File" where the MenuStrip says "Type Here", and type in "Edit" without the quotes in this Box, then in the Box below that type in "Cut", below that "Copy", then "Paste" and finally "Select All". The MenuStrip should look as below:
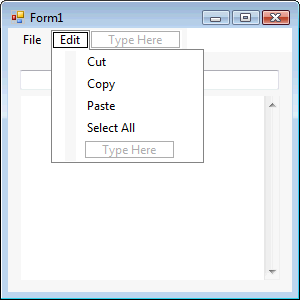
Step 14
Click or select next to "Edit" where the MenuStrip says "Type Here", and type in "View" without the quotes in this Box, then in the Box below that type in "Go To", below that "Home", then "Back", "Forward", "Refresh" and finally "Stop". The MenuStrip should look as below:
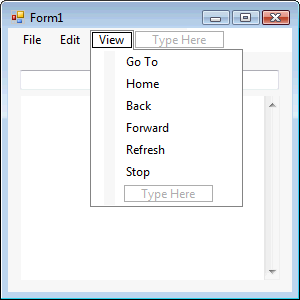
Step 15
Right Click on the Form or the entry of the Form in Solution Explorer and choose the "View Code" option then above the "Public Class frmMain" type the following:
Private Const BLOCK_DEFAULT As String = "[DEFAULT]" Private Const BLOCK_INTERNETSHORTCUT As String = "[InternetShortcut]" Private Const ITEM_URL As String = "URL=" Private Const ITEM_BASEURL As String = "BASEURL="
Also while still in the Code View, below the "Public Class frmMain" part type the following Subroutine:
Private Sub Navigate(ByRef URL As String) If String.IsNullOrEmpty(URL) Then Return If URL.Equals("about:blank") Then Return If Not URL.StartsWith("http://") And _ Not URL.StartsWith("https://") Then URL = "http://" & URL End If Try webMain.Navigate(URL) Catch ex As Exception ' Do nothing on Exception End Try End Sub
See Below:
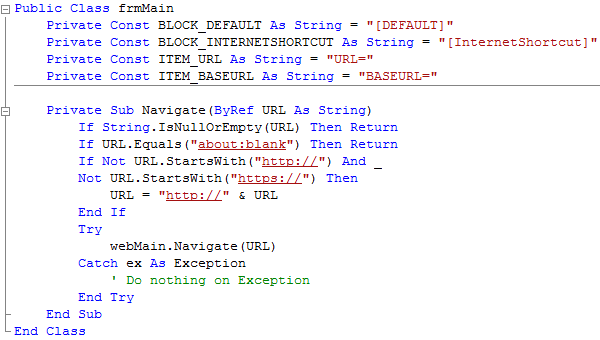
Step 16
Return to the Design view by selecting the [Design] tab or Right Click on the "View Designer" option in Solution Explorer for frmMain. Click on "File" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Open" (OpenToolStripMenuItem) and type the following in the OpenToolStripMenuItem_Click() Sub:
Dim Open As New OpenFileDialog() Dim URLFile As String() Dim myStreamReader As System.IO.StreamReader Open.Filter = "Internet Favourite (*.url)|*.url|All files (*.*)|*.*" Open.CheckFileExists = True Open.ShowDialog(Me) Try Open.OpenFile() myStreamReader = System.IO.File.OpenText(Open.FileName) URLFile = myStreamReader.ReadToEnd().Split(New String() {ControlChars.CrLf}, _ StringSplitOptions.RemoveEmptyEntries) For Each Item As String In URLFile If Item.StartsWith(ITEM_URL) Then Navigate(Item.Substring(ITEM_URL.Length)) Exit For End If Next Catch ex As Exception ' Do nothing on Exception End Try
See Below:
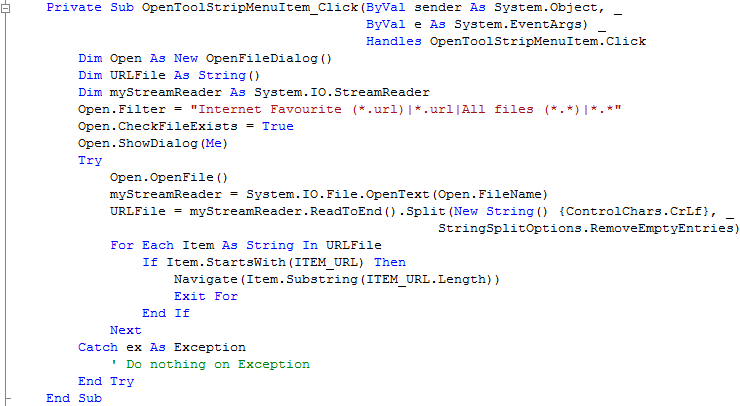
Step 17
Click on the [Design] Tab to view the form again, then Click on "File" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Save..." (SaveToolStripMenuItem) and type the following in the SaveToolStripMenuItem_Click() Sub
Dim Save As New SaveFileDialog() Dim myStreamWriter As System.IO.StreamWriter Save.Filter = "Internet Favourite (*.url)|*.url|All files (*.*)|*.*" Save.CheckPathExists = True Save.FileName = webMain.DocumentTitle Save.ShowDialog(Me) Try myStreamWriter = System.IO.File.CreateText(Save.FileName) myStreamWriter.Write(BLOCK_DEFAULT & vbCrLf & _ ITEM_BASEURL & webMain.Url.ToString & vbCrLf & _ BLOCK_INTERNETSHORTCUT & vbCrLf & _ ITEM_URL & webMain.Url.ToString) myStreamWriter.Flush() Catch ex As Exception ' Do nothing on Exception End Try
See Below:
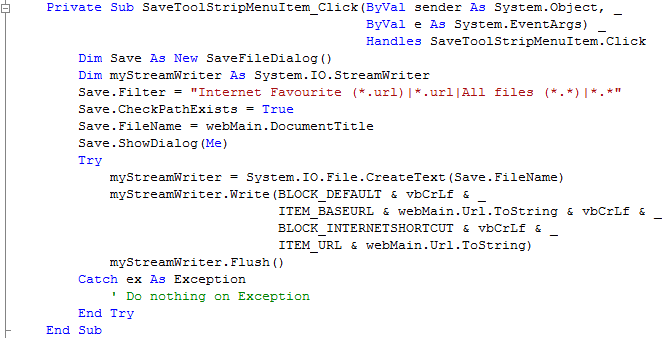
Step 18
Click on the [Design] Tab to view the form again, then Click on "File" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Exit" (ExitToolStripMenuItem) and type the following in the ExitToolStripMenuItem_Click() Sub
Dim Response As MsgBoxResult Response = MsgBox("Are you sure you want to Exit Web Browser?", _ MsgBoxStyle.Question + MsgBoxStyle.YesNo, _ "Web Browser") If Response = MsgBoxResult.Yes Then End End If
See Below:
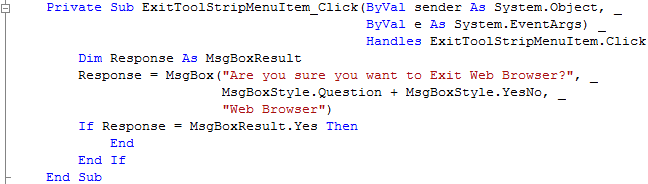
Step 19
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Cut" (CutToolStripMenuItem) and type the following in the CutToolStripMenuItem_Click() Sub
txtURL.Cut()
See Below:

Step 20
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Copy" (CopyToolStripMenuItem) and type the following in the CopyToolStripMenuItem_Click() Sub
txtURL.Copy()
See Below:

Step 21
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Paste" (PasteToolStripMenuItem) and type the following in the PasteToolStripMenuItem_Click() Sub
txtURL.Paste()
See Below:

Step 22
Click on the [Design] Tab to view the form again, then Click on "Edit" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Select All" (SelectAllToolStripMenuItem) and type the following in the SelectAllToolStripMenuItem_Click() Sub
txtURL.SelectAll()
See Below:

Step 23
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Go To" (GoToToolStripMenuItem) and type the following in the GoToToolStripMenuItem_Click() Sub
Navigate(txtURL.Text)
See Below:

Step 24
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Home" (HomeToolStripMenuItem) and type the following in the HomeToolStripMenuItem_Click() Sub
webMain.GoHome()
See Below:

Step 25
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Back" (BackToolStripMenuItem) and type the following in the BackToolStripMenuItem_Click() Sub
webMain.GoBack()
See Below:

Step 26
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Forward" (ForwardToolStripMenuItem) and type the following in the ForwardToolStripMenuItem_Click() Sub
webMain.GoForward()
See Below:

Step 27
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Refresh" (RefreshToolStripMenuItem) and type the following in the RefreshToolStripMenuItem_Click() Sub
If Not webMain.Url.Equals("about:blank") Then webMain.Refresh() End If
See Below:

Step 28
Click on the [Design] Tab to view the form again, then Click on "View" on the MenuStrip to show the MenuStrip then Double Click on the Menu Item Labeled "Stop" (StopToolStripMenuItem) and type the following in the StopToolStripMenuItem_Click() Sub
webMain.Stop()
See Below:

Step 29
Click on the [Design] Tab to view the form again, then Double Click on the Form (frmMain) and type the following in the frmMain_Load() Sub
Me.Text = "Web Browser"
See Below:

Step 30
While Still in the Code View, if not double click on the Form, the Window Area of the Form will work, this will show the frmMain_Load Sub from the previous step, on this screen at the top are two Drop-down boxes one labeled (frmMain Events) and the other is Load. Click on the drop-down box with "(frmMain Events)" in it and select "txtURL", then from the list "(Declarations)" select the KeyDown option from the list of events for txtURL, and type in txtURL_KeyDown() Sub
If (e.KeyCode = Keys.Enter) Then Navigate(txtURL.Text) End If
See Below:

Step 31
Again while Still in the Code View with the two combo boxes (General) and (Declarations), select "webMain" from the first list and then from the list "(Declarations)" select the "Navigated" ooption from the list of events for webMain, and type in webMain_Navigated() Sub
Me.Text = "Web Browser - " & webMain.DocumentTitle txtURL.Text = webMain.Url.ToString
See Below:

Step 32
Steps 31-36 are optional and just add Keyboard Shortcuts to the MenuItems, you don't have to do these if you don't want to!
Return to the Design view of the Form by selecting the [Design] tab for the Form, or double click on the Form's name in Solution Explorer.
Click on "File" on the Menu Strip to select the Menu Strip and show the File Menu then mouse over the "Type Here" box.
Click on the Drop Down arrow inside the "Type Here" box to show the following options: MenuItem, ComboBox, Separator and TextBox, see below:
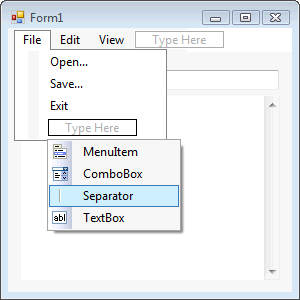
Step 33
Click on the "Separator" option to place a Menu Separator in this menu, then click on it an move it so it goes above the "Exit" option in the file menu, do this by clicking on the separator (the horizontal line which has appeared) then whilst keeping clicked move it up, it should appear as below:
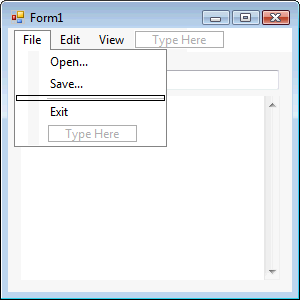
Step 34
With the "File" Menu still being displayed click on "Open" then in the Properties box look for the "Shortcut Key" option and click on the Drop Down arrow where "None" appears. Check the "Ctrl" Checkbox in "Modifiers" and then in the "Key" dropdown list select "O", see below:
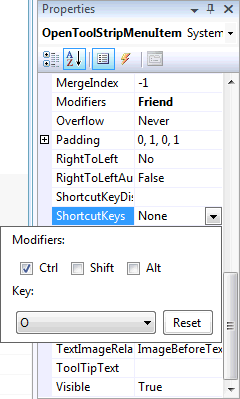
Step 35
Again with the "File" menu displayed click on "Save" and set the "Shortcut Key" to "Ctrl" and "S", the File Menu should appear as below:
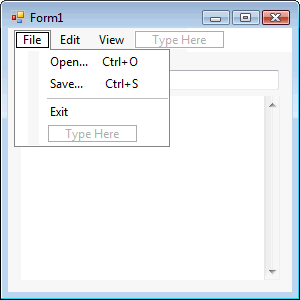
Step 36
Click on or select the "Edit" Menu and set the "Shortcut Keys" Properties, "Cut" should be set to "Ctrl" and "X", "Copy" to "Ctrl" and "C", "Paste" to "Ctrl" and "V", "Select All" should be "Ctrl" and "A". The Edit Menu should appear as below:
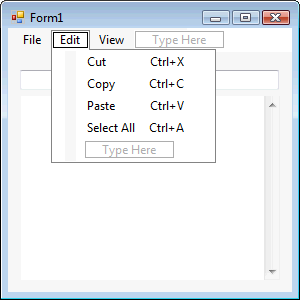
Step 37
Click on or select the "View" Menu, select "Go To" and in the "ShortcutKeys" property type "Ctrl+Enter", select "Home" and type "Ctrl+Home" in the "ShortcutKeys" property. "Back" should be set to "Ctrl" and "Left", "Forward" should be "Ctrl" and "Right" and "Refresh" should be just "F5". The View Menu should appear as below:
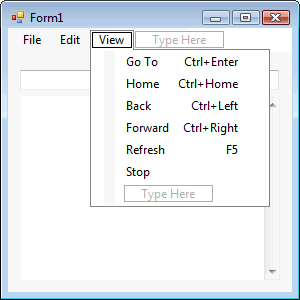
Step 38
Save the Project as you have now finished the application, then click on Start:

When you do the following will appear:
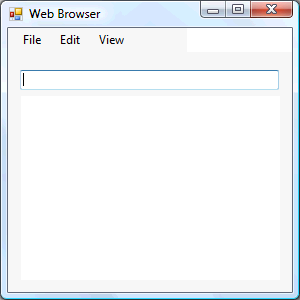
Step 39
You can type into the TextBox any website address then click on Go To or just press Enter/Ctrl+Enter to visit that website, you can Save this address to open later, see below:
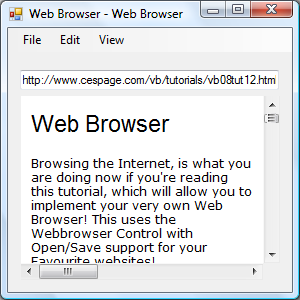
Step 40
Click File then Exit or click on the Close button
on the top right of Web Browser to end the application.
This program uses the standard URL format to Save the Website and the WebBrowser control to display it, this control supports other features such as Printing and Saving the Webpage itself to see what other functions it supports type "webMain." without the quotes, and the functions supported will be shown!