Noughts and Crosses or Tic-Tac-Toe, what ever you waht call it, a very simple game to play. This Tutorial features drawing graphics, message boxes, arrays, pictureboxes and more!
Printer Friendly Download Tutorial (199KB) Download Source Code (15.1KB)
Step 1
Start Microsoft Visual Basic 2005 Express Edition, then select File then New Project... Choose Windows Application from the New Project Window, enter a name for the Project and then click OK, see below:
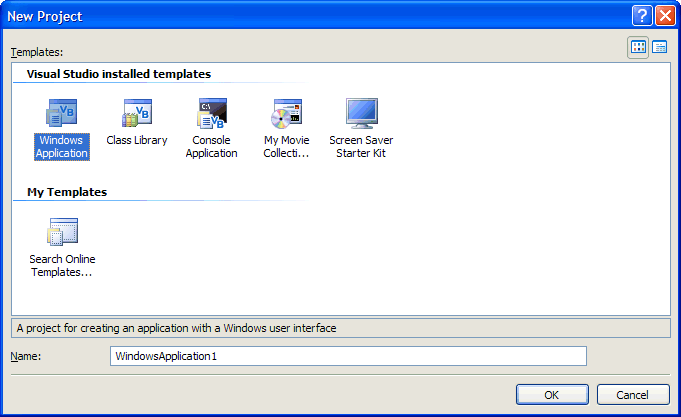
Step 2
A Blank Form named Form1 should then appear, see below:
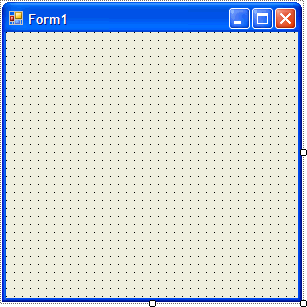
Step 3
Then from the Windows Forms components tab select the PictureBox control:
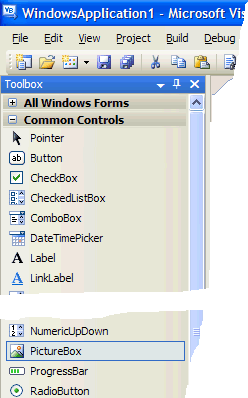
Step 4
Draw Nine PictureBoxes of 60x60 pixels in Size on the Form and in the Order indicated, PictureBox1 is 1, PictureBox2 is 2 etc, see below:
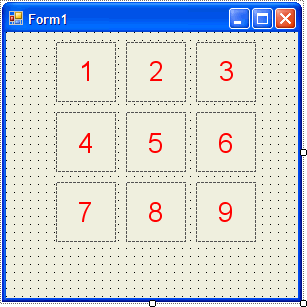
Step 5
Select each PictureBox (PictureBox1 to PictureBox9) and then go to the Properties box and change the BackColor property from Control to White, see below:
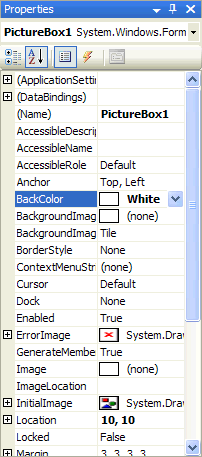
Step 6
Then from the Windows Forms components tab select the Button control:
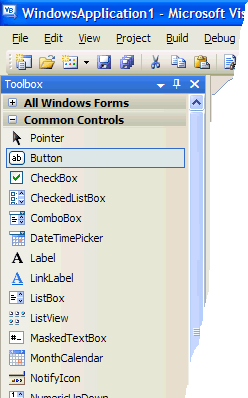
Step 7
Draw a Button on the Form and set the Text Property to be New Game in the Properties box so the form looks as below:
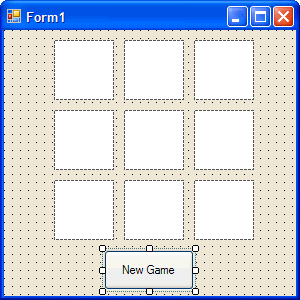
Step 8
Right click on the Form and select the View Code option then in the code view type in below Public Class Form1 the following:
Dim DrawPicture As New PictureBox Dim DrawBitmap As Bitmap Dim DrawGraphics As Graphics Dim CirclePen As New Pen(Color.Blue, 4) Dim CrossPen As New Pen(Color.Red, 4) Dim IsCross As Boolean Dim Nought As String = "O" Dim Cross As String = "X" Dim Won As Boolean Dim Board(3, 3) As String
See Below:
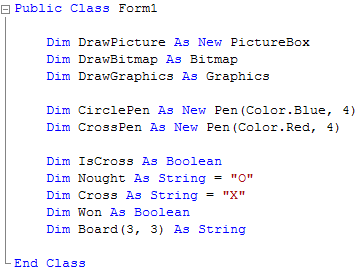
Step 9
While still in the Code view type below the Declarations entered in the previous step (anything starting with Dim) the following:
Private Sub DrawX() DrawGraphics.SmoothingMode = Drawing2D.SmoothingMode.HighQuality DrawGraphics.DrawLine(CrossPen, 10, 10, 50, 50) DrawGraphics.DrawLine(CrossPen, 50, 10, 10, 50) End Sub Private Sub DrawO() DrawGraphics.SmoothingMode = Drawing2D.SmoothingMode.HighQuality DrawGraphics.DrawEllipse(CirclePen, 8, 8, 40, 40) End Sub
See Below:
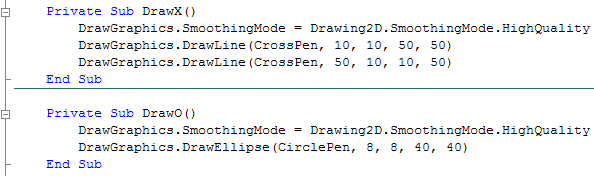
Step 10
After the DrawO() Sub has been typed in, type below it the following Method:
Private Sub SetPiece(ByVal Row As Integer, _ ByVal Column As Integer, ByVal pic As PictureBox) If Board(Row, Column) = Nought Or Board(Row, Column) = Cross Then ' Do nothing if Nought or Cross already in square Else DrawPicture = CType(pic, PictureBox) DrawBitmap = New Bitmap(DrawPicture.Width, DrawPicture.Height) DrawGraphics = Graphics.FromImage(DrawBitmap) DrawPicture.Image = DrawBitmap If IsCross Then DrawX() Board(Row, Column) = Cross Me.Text = "Player:" & Nought Else DrawO() Board(Row, Column) = Nought Me.Text = "Player:" & Cross End If End If End Sub
See Below:
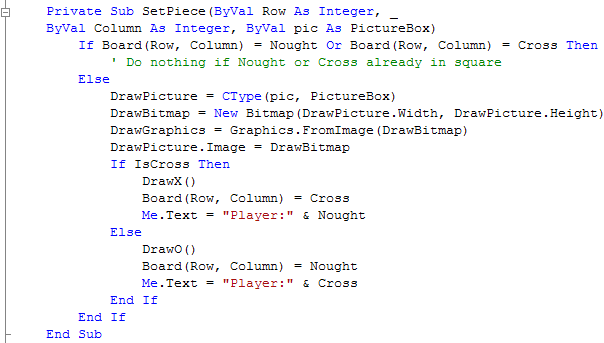
Step 11
After the SetPiece() Sub type in the following Win and Draw Checking routines:
Private Sub Check(ByVal Player As String, ByVal x1 As Integer, ByVal y1 As Integer, _ ByVal x2 As Integer, ByVal y2 As Integer, ByVal x3 As Integer, ByVal y3 As Integer) If Board(x1, y1) = Player And Board(x2, y2) = Player And Board(x3, y3) = Player Then Won = True Me.Text = Player & " Won!" MsgBox(Player & " Won!", MsgBoxStyle.Information, "Noughts and Crossses") End If End Sub Private Sub CheckWon(ByVal Player As String) Check(Player, 1, 1, 1, 2, 1, 3) Check(Player, 2, 1, 2, 2, 2, 3) Check(Player, 3, 1, 3, 2, 3, 3) Check(Player, 1, 1, 2, 1, 3, 1) Check(Player, 1, 2, 2, 2, 3, 2) Check(Player, 1, 3, 2, 3, 3, 3) Check(Player, 1, 1, 2, 2, 3, 3) Check(Player, 3, 1, 2, 2, 1, 3) End Sub Private Sub CheckDraw() If Not Board(1, 1) = "" And Not Board(1, 2) = "" And Not Board(1, 3) = "" Then If Not Board(2, 1) = "" And Not Board(2, 2) = "" And Not Board(2, 3) = "" Then If Not Board(3, 1) = "" And Not Board(3, 2) = "" And Not Board(3, 3) = "" Then If Not Won Then Me.Text = "Draw!" MsgBox("Draw!", MsgBoxStyle.Information, "Noughts and Crossses") End If End If End If End If End Sub
See Below:
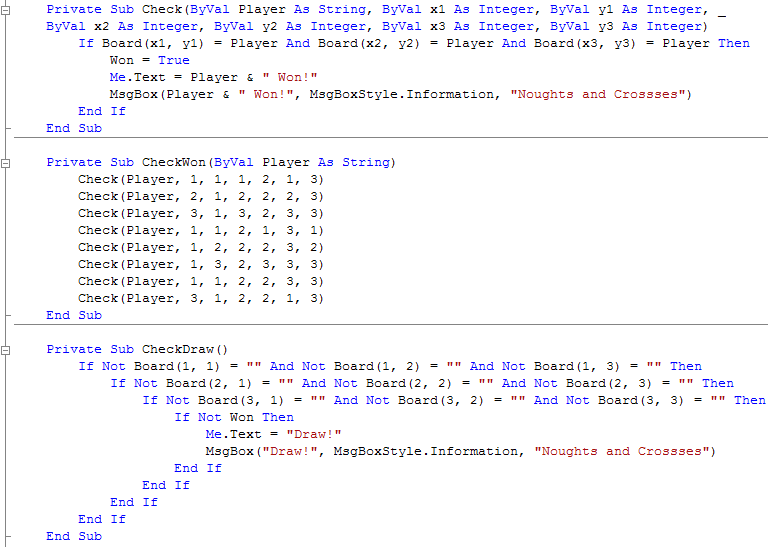
Step 12
After the CheckDraw() Sub type in the following PictureBox Click handler method:
Private Sub PictureClick(ByVal sender As System.Object, _ ByVal e As System.EventArgs) Handles PictureBox1.Click, _ PictureBox2.Click, PictureBox3.Click, PictureBox4.Click, _ PictureBox5.Click, PictureBox6.Click, PictureBox7.Click, _ PictureBox8.Click, PictureBox9.Click If Not Won Then Select Case sender.name Case "PictureBox1" SetPiece(1, 1, sender) Case "PictureBox2" SetPiece(1, 2, sender) Case "PictureBox3" SetPiece(1, 3, sender) Case "PictureBox4" SetPiece(2, 1, sender) Case "PictureBox5" SetPiece(2, 2, sender) Case "PictureBox6" SetPiece(2, 3, sender) Case "PictureBox7" SetPiece(3, 1, sender) Case "PictureBox8" SetPiece(3, 2, sender) Case "PictureBox9" SetPiece(3, 3, sender) End Select CheckWon(Cross) CheckWon(Nought) CheckDraw() IsCross = Not IsCross Else MsgBox("Game is Over") End If End Sub
See Below:
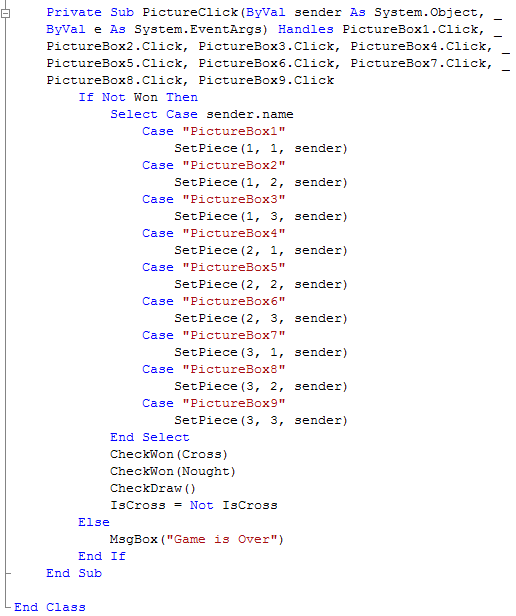
Step 13
Right click on the Code View and select the View Designer option then Double Click on the Button and type the following in Button1_Click method:
Dim msg As MsgBoxResult Board(1, 1) = "" Board(1, 2) = "" Board(1, 3) = "" Board(2, 1) = "" Board(2, 2) = "" Board(2, 3) = "" Board(3, 1) = "" Board(3, 2) = "" Board(3, 3) = "" PictureBox1.Image = Nothing PictureBox2.Image = Nothing PictureBox3.Image = Nothing PictureBox4.Image = Nothing PictureBox5.Image = Nothing PictureBox6.Image = Nothing PictureBox7.Image = Nothing PictureBox8.Image = Nothing PictureBox9.Image = Nothing Won = False msg = MsgBox(Cross & " to go first?", _ MsgBoxStyle.Question + MsgBoxStyle.YesNo, "Noughts and Crossses") If msg = MsgBoxResult.Yes Then IsCross = True Me.Text = "Player:" & Cross Else IsCross = False Me.Text = "Player:" & Nought End If
See Below:
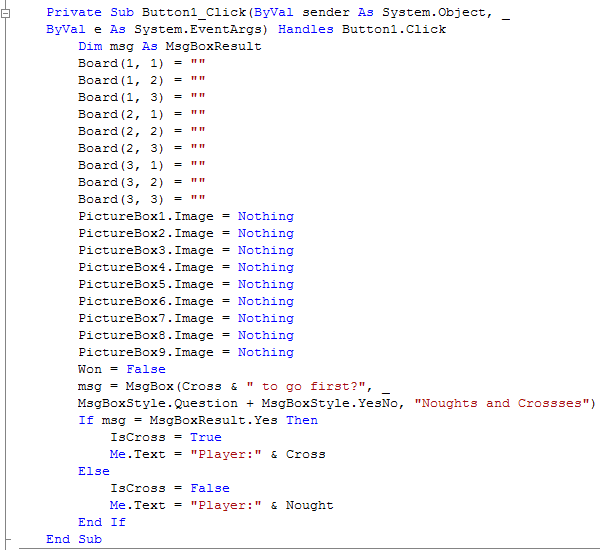
Step 14
Right click on the Code View again and select the View Designer option then Double Click on the Form and type the following in the Form1_Load method:
Button1.PerformClick()
See Below:

Step 15
Save the Project as you have now finished the application, then click on Start:

When you do the question should X go first will appear choose Yes or Now then the following will appear:
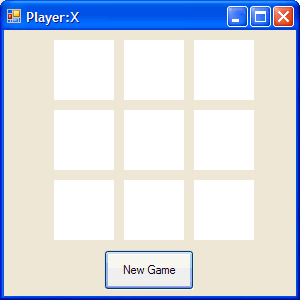
Step 16
Click on one of the Picture boxes to place a O or an X continue until you Win, Lose or Draw, see below:
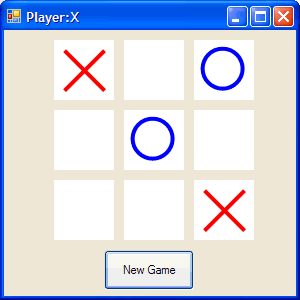
Step 17
Click on the Close button on the top right of Form1 to end the application.
That was another great game created using Visual Basic 2005 Express Edition, feel free to amend the game you might even want to write a Computer Player 2 so you can try and beat the computer using random numbers or change the colours of the noughts and crosses.