Slide Show is a Silverlight-based JPEG Image Viewer with support for Play/Pause Slideshow with Playback control such as Speed and more!
Printer Friendly Download Tutorial (628KB) Download Source Code (8.41KB) Online Demonstration
Step 1
Start Microsoft Visual Web Developer 2010 Express, then Select File then New Project... Select "Visual Basic" then "Silverlight Application" from Templates, select a Location if you wish, then enter a name for the Project and then click OK, see below:
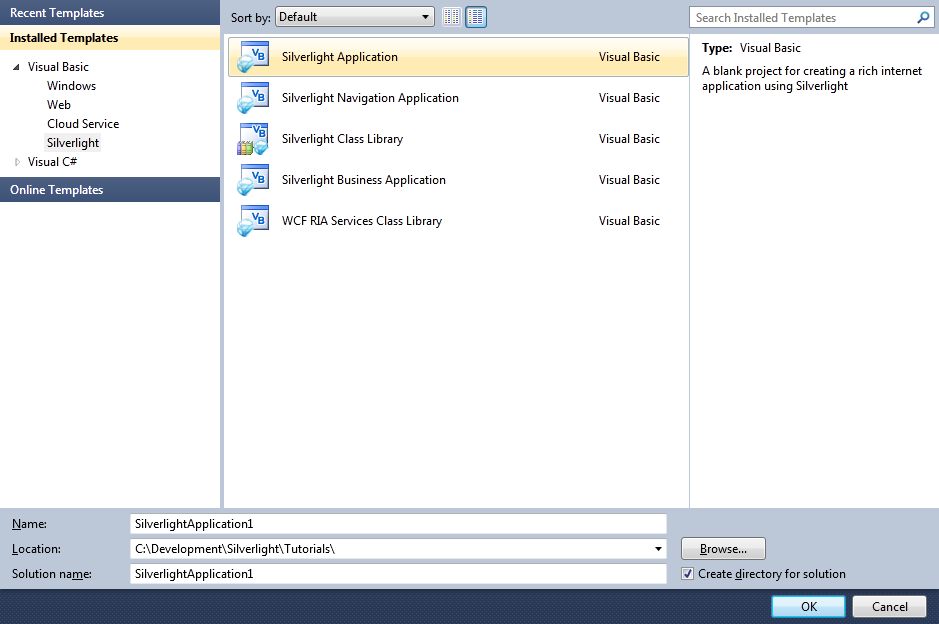
Step 2
New Silverlight Application window should appear, uncheck the box "Host the Silverlight Application in a new Web site" and then select the required Silverlight Version, see below:
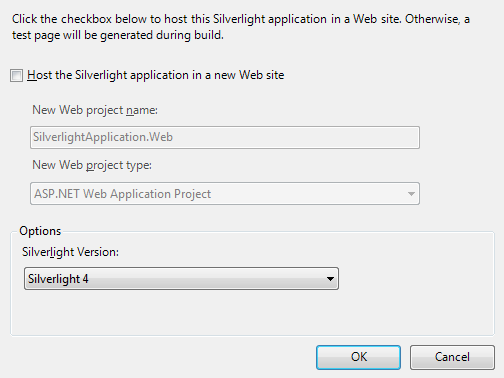
Step 3
A Blank Page named MainPage.xaml should then appear, see below:
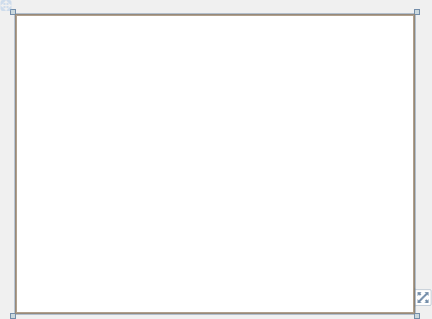
Step 4
Select Project then Properties... The "Properties" window should appear, check the box "Enable running application out of the browser", see below:
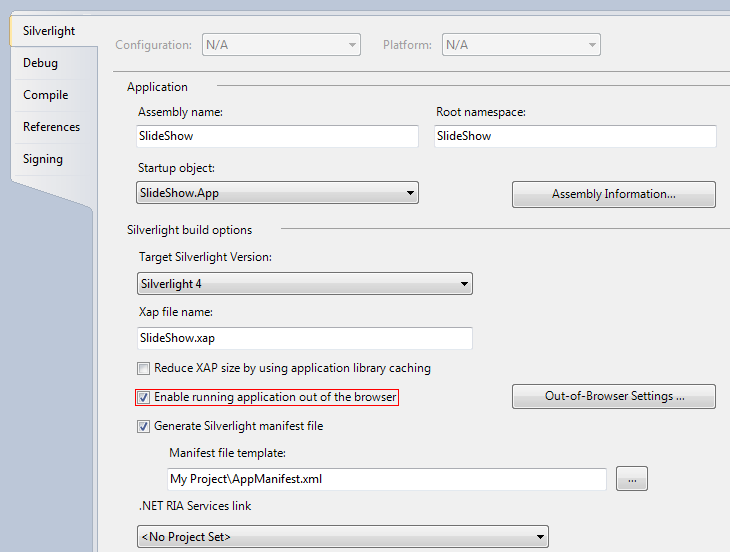
Step 5
While still in the Properties Window, click on the "Out-of-Browser Settings..." button, set the "Width" value to 400 and the "Height" to 300,
then check the box "Require elevated trust when running outside the browser", see below:
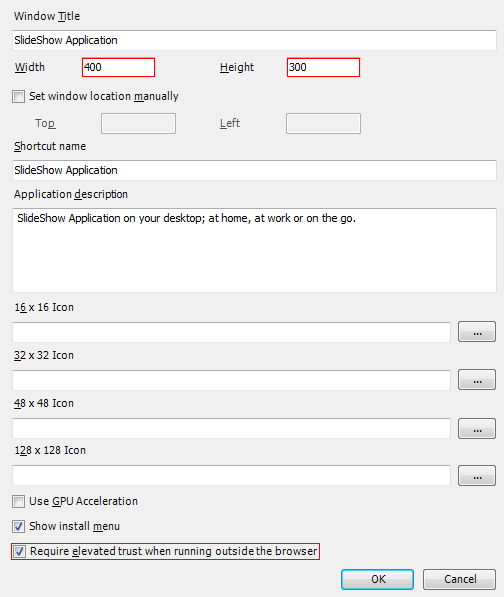
Step 6
Confirm the Settings by Clicking on OK.
Select Project then "Add Class...", and select the "Class" Template if not already Selected, then change the "Name" to SlideShow.vb , see below:
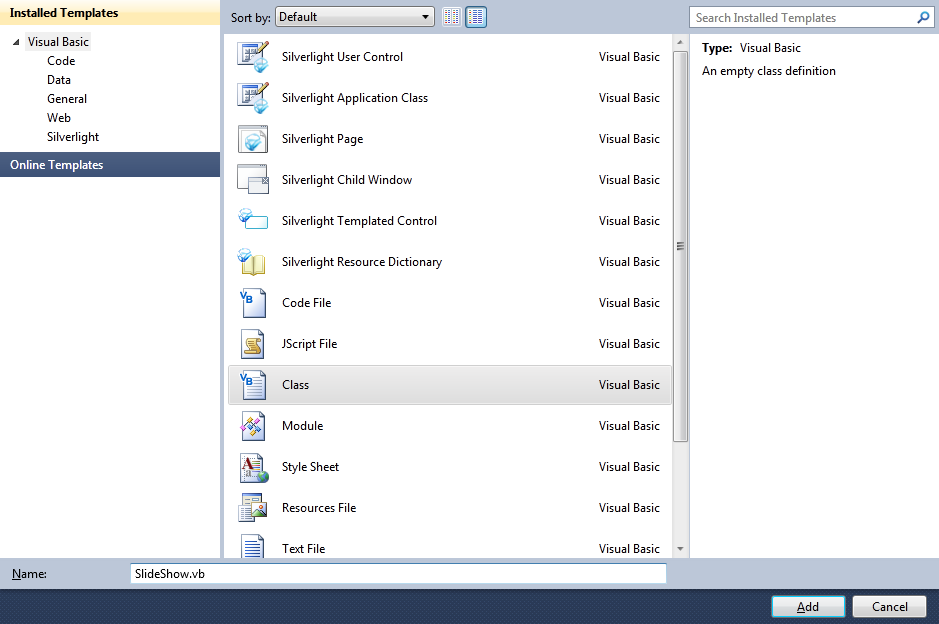
Step 7
Add the new Class to the Project by Clicking on Add, then in the Code View for the SlideShow.vb Class, above the "Public Class SlideShow" line type the following:
Imports System.Windows.Media.Imaging
See Below:
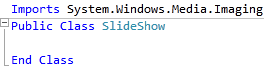
Step 8
While still in the Code View for the SlideShow.vb Class, below the "Public Class SlideShow" line type the following:
Private WithEvents _slideshow As New Windows.Threading.DispatcherTimer Private _images As New List(Of BitmapImage) Private _index As Integer Private _hasImages As Boolean Private _isPlaying As Boolean Private _isPaused As Boolean Private _isRepeat As Boolean Public Event Slide(ByVal Image As BitmapImage, ByVal Index As Integer)
See Below:
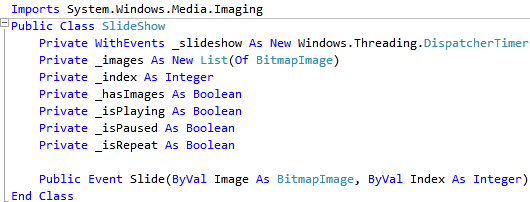
Step 9
While still in the Code View the SlideShow Class, above the "End Class" for "Public Class SlideShow", type the following Constructor and Function:
''' <summary>Constructor</summary> ''' <remarks>Initialise Values</remarks> Public Sub New() _slideshow.Interval = TimeSpan.FromSeconds(1.5) End Sub ''' <summary>Browse</summary> ''' <param name="Path">Directory to Browse</param> ''' <returns>True if Success, False if no JPEGs Found</returns> ''' <remarks>Requires Full Trust</remarks> Public Function Browse(ByRef Path As String) As Boolean Dim _dirInfo As New IO.DirectoryInfo(Path) _images.Clear() For Each File In _dirInfo.EnumerateFiles("*.j*pg") Using FileStream As IO.Stream = File.OpenRead Dim Source As New BitmapImage Source.SetSource(FileStream) _images.Add(Source) End Using Next _hasImages = _images.Count > 0 Return _hasImages End Function
See Below:
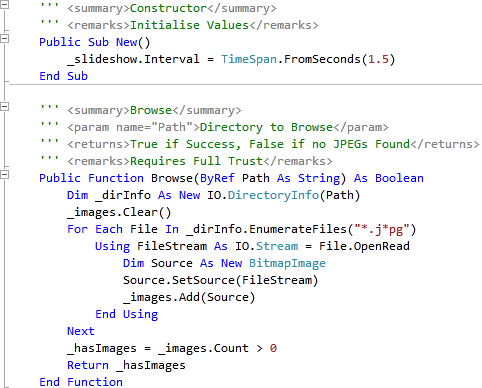
Step 10
While still in the Code View the SlideShow Class, above the "End Class" for "Public Class SlideShow", type the following Subs:
''' <summary>Play</summary> ''' <remarks>Play SlideShow</remarks> Public Sub Play() If _hasImages Then If _isPaused Or Not _isPlaying Then _slideshow.Start() _isPlaying = True _isPaused = False End If End If End Sub ''' <summary>Pause</summary> ''' <remarks>Pause SlideShow</remarks> Public Sub Pause() If _hasImages Then If _isPlaying Then _slideshow.Stop() _isPaused = True _isPlaying = False End If End If End Sub ''' <summary>Stop</summary> ''' <remarks>Stop SlideShow</remarks> Public Sub [Stop]() If _hasImages Then _index = 0 _slideshow.Stop() _isPaused = False _isPlaying = False End If End Sub ''' <summary>Selected</summary> ''' <param name="Index">Selected Index</param> ''' <remarks>Selected Slide</remarks> Public Sub Selected(ByRef index As Integer) If _hasImages Then If index < _images.Count Then _index = index If _isPlaying Then Me.Play() End If End If End If End Sub ''' <summary>SlideShow_Tick</summary> ''' <param name="sender">Object</param> ''' <param name="e">Event</param> ''' <remarks>SlideShow DispatcherTimer</remarks> Private Sub SlideShow_Tick(ByVal sender As Object, _ ByVal e As EventArgs) _ Handles _slideshow.Tick If _hasImages And _isPlaying Then If _index < _images.Count Then RaiseEvent Slide(_images.Item(_index), _index) _index += 1 Else If _isRepeat Then _index = 0 ' Reset if Repeat Else Me.Stop() ' Stop End If End If End If End Sub
See Below:
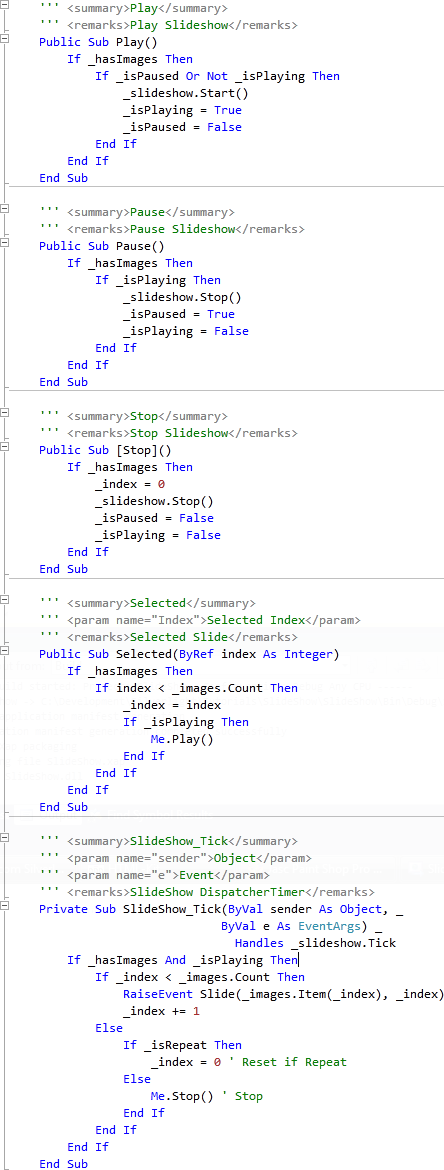
Step 11
While still in the Code View for SlideShow.vb, above the "End Class" for "Public Class SlideShow", type the following Properties:
''' <summary>Speed</summary> ''' <value>TimeSpan</value> ''' <returns>DispatchTimer TimeSpan</returns> ''' <remarks>SlideShow Interval (Speed)</remarks> Public Property Speed As TimeSpan Get Return _slideshow.Interval End Get Set(ByVal value As TimeSpan) If value <> _slideshow.Interval Then _slideshow.Interval = value End If End Set End Property ''' <summary>Position</summary> ''' <value>Integer</value> ''' <returns>Integer</returns> ''' <remarks>SlideShow Current Position</remarks> Public Property Position As Integer Get Return _index End Get Set(ByVal value As Integer) _index = value End Set End Property ''' <summary>Count</summary> ''' <value>Integer</value> ''' <returns>Images List(Of BitmapImage) Count</returns> ''' <remarks>SlideShow Count</remarks> Public ReadOnly Property Count As Integer Get Return _images.Count End Get End Property ''' <summary>Playing</summary> ''' <value>Boolean</value> ''' <returns>True if Playing, False if Not</returns> ''' <remarks>SlideShow Playing</remarks> Public ReadOnly Property Playing As Boolean Get Return _isPlaying End Get End Property ''' <summary>Paused</summary> ''' <value>Boolean</value> ''' <returns>True if Paused, False if Not</returns> ''' <remarks>SlideShow Paused</remarks> Public ReadOnly Property Paused As Boolean Get Return _isPaused End Get End Property ''' <summary>Repeat</summary> ''' <value>Boolean</value> ''' <returns>Boolean</returns> ''' <remarks>Repeat SlideShow</remarks> Public Property Repeat() As Boolean Get Return _isRepeat End Get Set(ByVal value As Boolean) _isRepeat = value End Set End Property
See Below:
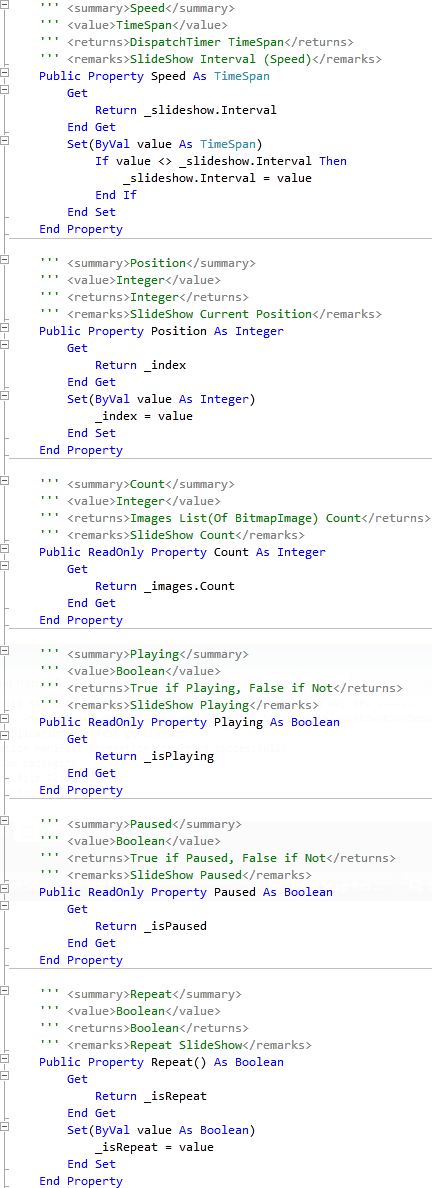
Step 12
Return to the MainPage Designer View by selecting the "MainPage.xaml" Tab.
Then from the All Silverlight Controls section in the Toolbox select the Canvas control:
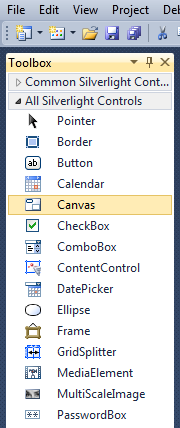
Step 13
Draw a Two Canvases on the Page then in the XAML Pane above the "</Grid>" then change the "<Canvas>" lines to the following:
<Canvas Height="35" Width="400" VerticalAlignment="Top" HorizontalAlignment="Left" Name="Toolbar"></Canvas> <Canvas Height="35" Width="400" Margin="0,265,0,0" VerticalAlignment="Top" HorizontalAlignment="Left" Name="Playback"></Canvas>
See below:
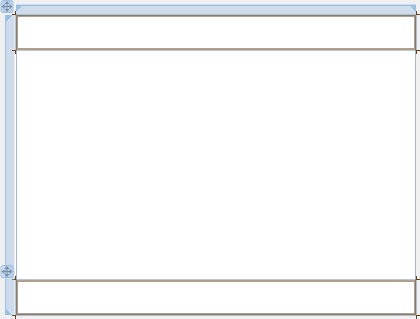
Step 14
Then from the Common Silverlight Controls section in the Toolbox select the Button control:
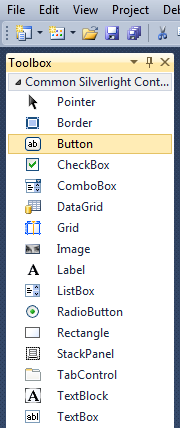
Step 15
Draw Three Buttons on the "Toolbar" Canvas by dragging the Buttons from the Toolbox onto the Canvas then in the XAML Pane inbetween top the "<Canvas>" and "</Canvas>" tags change the "<Button>" lines to the following:
<Button Canvas.Left="6" Canvas.Top="6" Height="23" Width="75" Name="Browse" Content="Browse..."/> <Button Canvas.Left="87" Canvas.Top="6" Height="23" Width="75" Name="PlayPause" Content="Play Pause"/> <Button Canvas.Left="168" Canvas.Top="6" Height="23" Width="75" Name="Stop" Content="Stop"/>
See below:
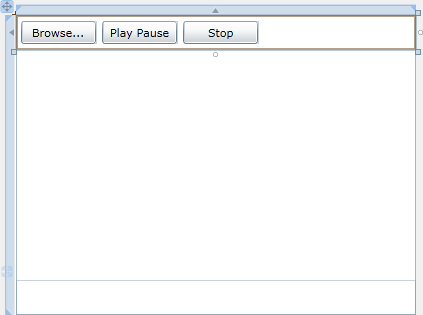
Step 16
Then from the Common Silverlight Controls section in the Toolbox select the CheckBox control:
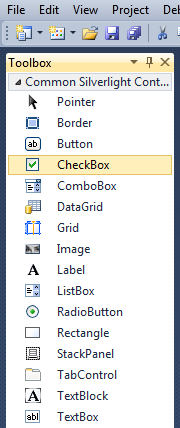
Step 17
Draw a CheckBox on the Upper Canvas (Toolbar) next to the Buttons, and change the <CheckBox> line to the following:
<CheckBox Canvas.Left="249" Canvas.Top="10" Height="17" Width="75" Content="Repeat" Name="Repeat"/>
See below:
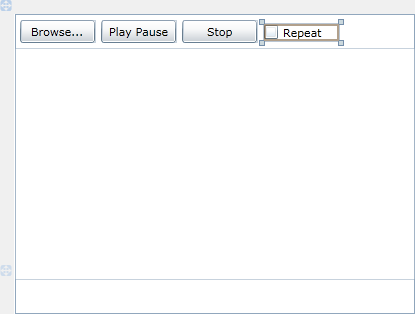
Step 18
Then from the All Silverlight Controls section in the Toolbox select the Slider control:
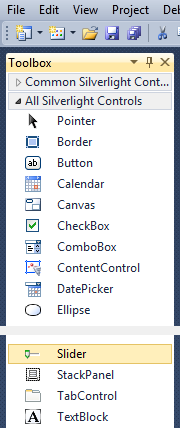
Step 19
Draw Two Sliders onto the Lower Canvas (Playback) and change the <Slider> lines to the following:
<Slider Canvas.Left="6" Canvas.Top="6" Height="23" Width="237" Name="Position"/> <Slider Canvas.Left="249" Canvas.Top="6" Height="23" Width="75" Minimum="100" Maximum="5000" Value="1500" Name="Speed"/>
See below:
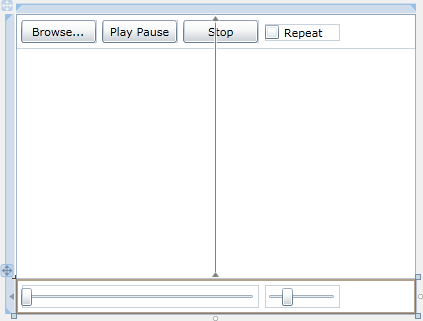
Step 20
Then from the Common Silverlight Controls section in the Toolbox select the Image control:
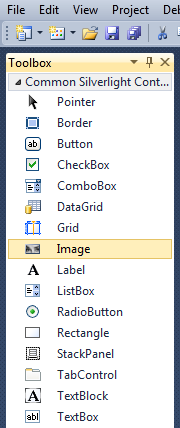
Step 21
Draw an Image on the Page between the Canvases and in the XAML Pane change the "<Image>" line to the following:
<Image Height="230" Width="400" Margin="0,35,0,0" HorizontalAlignment="Left" VerticalAlignment="Top" Stretch="Uniform" Name="Display"/>
See below:
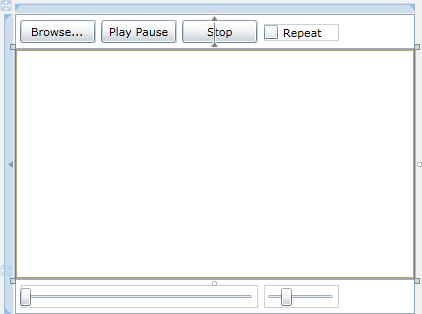
Step 22
Right Click on the Page or the entry for "MainPage.xaml" in Solution Explorer and choose the "View Code" option. In the Code View below the "Inherits UserControl" line type the following:
Public WithEvents SlideShow As New SlideShow
See Below:
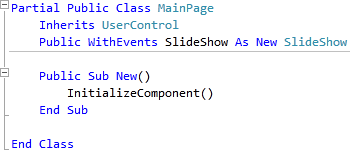
Step 23
While still in the Code View, below the "End Sub" for "Public Sub New()" Constructor type the following Event Handler Sub:
Private Sub SlideShow_Slide(ByVal Image As Imaging.BitmapImage, _ ByVal Index As Integer) _ Handles SlideShow.Slide Display.Source = Image Position.Value = Index End Sub
See Below:

Step 24
Return to the Designer View by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Browse..." Button and type in the Browse_Click Sub:
Dim OpenDialog As New OpenFileDialog OpenDialog.Filter = "JPEG Images (*.jpg;*.jpeg)|*.jpg;*.jpeg" If OpenDialog.ShowDialog Then Try If OpenDialog.File.Exists Then If App.Current.HasElevatedPermissions Then ' Show All Images If SlideShow.Browse(OpenDialog.File.DirectoryName) Then Position.Value = 0 Position.Minimum = 0 Position.Maximum = SlideShow.Count - 1 SlideShow.Play() End If Else ' Show Single Image Using FileStream As IO.Stream = OpenDialog.File.OpenRead Dim Source As New Imaging.BitmapImage Source.SetSource(FileStream) Display.Source = Source End Using End If End If Catch ex As Exception ' Ignore Errors End Try End If
See Below:
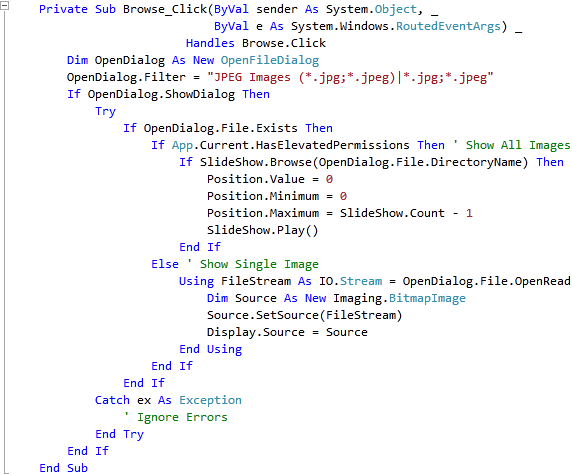
Step 25
Return to the Designer View by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Play Pause" Button and type in the PlayPause_Click Sub:
If SlideShow.Playing Then If SlideShow.Paused Then SlideShow.Play() Else SlideShow.Pause() End If Else SlideShow.Play() End If
See Below:
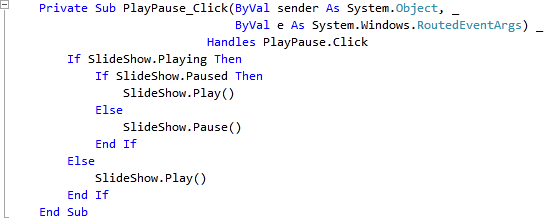
Step 26
Return to the Designer View by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Stop" Button Control and type in the Stop_Click Sub:
SlideShow.Stop()
See Below:

Step 27
Return to the Designer View by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Click on the "Repeat" Checkbox, then goto the Properties box and Click on the "Events" Tab, see below:
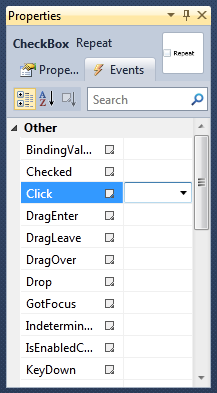
Double Click on the "Click" Event and type in the Repeat_Click Sub:
SlideShow.Repeat = Repeat.IsChecked
See Below:

Step 28
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Position" Slider Control, which is the larger Slider on the "Playback" Canvas, and type in the Position_ValueChanged Sub:
SlideShow.Position = CInt(Position.Value)
See Below:

Step 29
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Speed" Slider Control, which is next to the "Position" Slider, and type in the Speed_ValueChanged Sub:
SlideShow.Speed = TimeSpan.FromMilliseconds(Speed.Value)
See Below:

Step 30
Save the Project as you have now finished the Silverlight application. Select Debug then Start Debugging or click on Start Debugging:

After you do, the following will appear in a new Web Browser window:
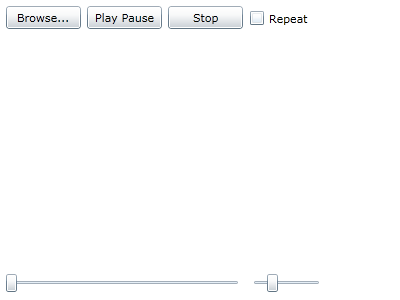
Step 31
If you Click on Browse... then Select a File, that Image will be Displayed, see below:
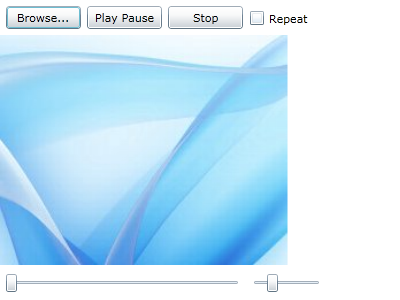
Step 32
Notice that this is not a Slide Show, this is because this feature only works Out-of-Browser with Full Trust to get all the Images.
Right-click on the Application in the Browser and choose the "Install SlideShow Application onto this Computer..." option to show the Installation Screen, see below:
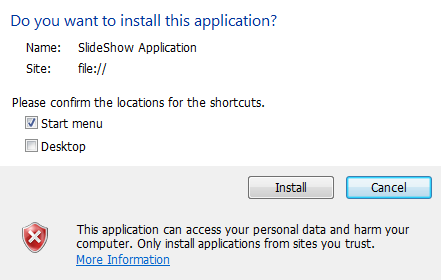
Step 33
Check the "Start menu" or "Desktop" boxes to add a Shortcut to the Application in either or both locations, and then click on the "Install" button, the following will then appear in a new Window:
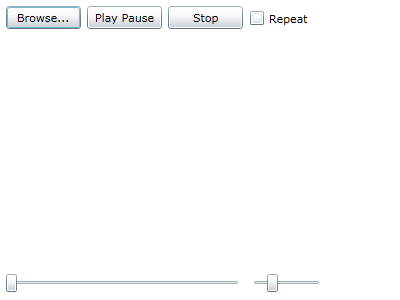
Step 34
Now if you Click on Browse... and Select a File, all the Images in the Same Folder will become a SlideShow, and start Playing, see below:
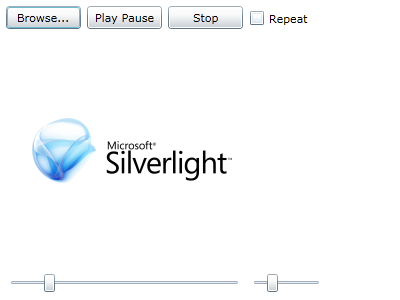
Step 35
Close the Application and Browser window by clicking on the Close Button on
the top right of the Application Window and Web Browser to Stop the application.
This is a Simple Slide Show application, you can try adding your own features such as Animations and Transitions between the Image or add other features, make it your own!