Create a Task List application with this Tutorial for Listbox-based To Do lists with Open/Save support using LINQ to XML plus Add/Remove Items.
Printer Friendly Download Tutorial (290KB) Download Source Code (6.76KB) Online Demonstration
Step 1
Start Microsoft Visual Web Developer 2010 Express, then Select File then New Project... Select "Visual Basic" then "Silverlight Application" from Templates, select a Location if you wish, then enter a name for the Project and then click OK, see below:
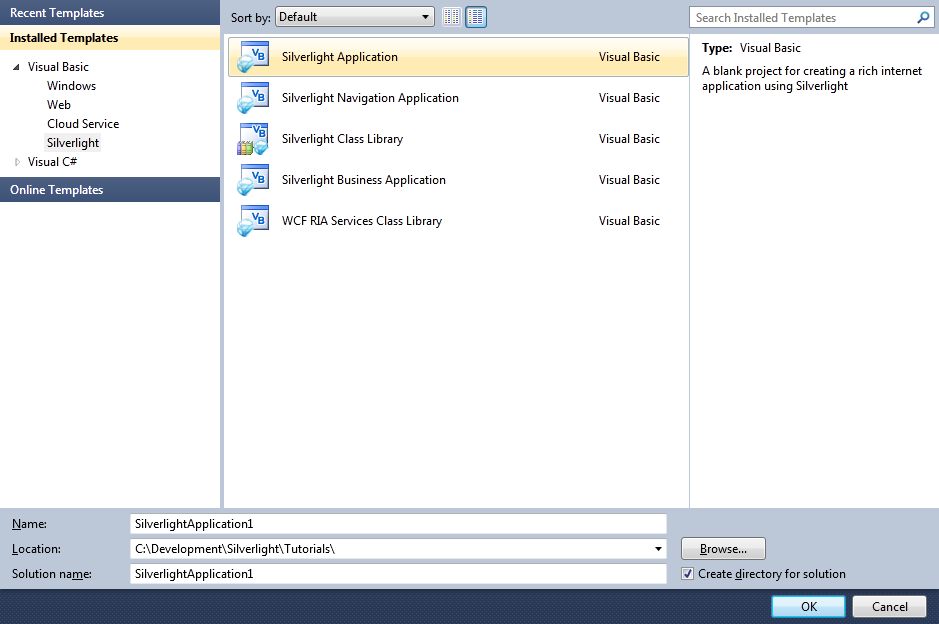
Step 2
New Silverlight Application window should appear, uncheck the box "Host the Silverlight Application in a new Web site" and then select the required Silverlight Version, see below:
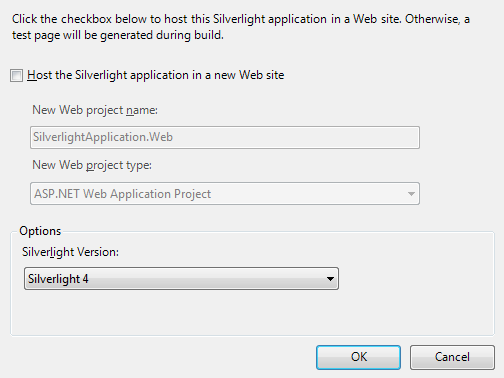
Step 3
A Blank Page named MainPage.xaml should then appear, see below:
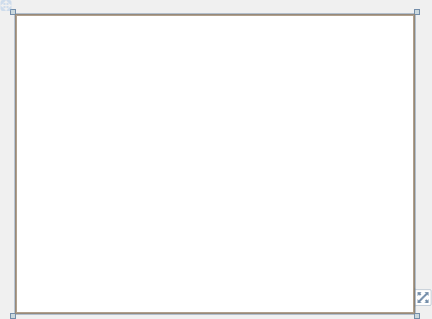
Step 4
Select Project then Add Reference... The "Add Reference" window should appear, select "System.XML.Linq" from the ".NET" List, see below:
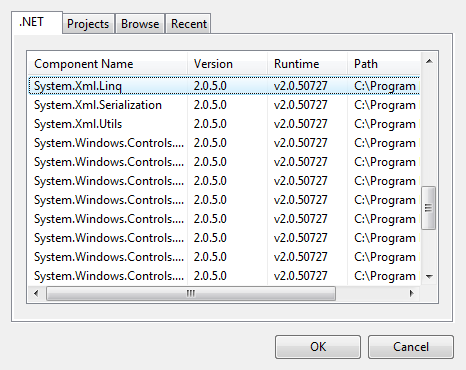
Step 5
Add the Reference to "System.XML.Linq" by Clicking on OK
Then from the All Silverlight Controls section in the Toolbox select the Canvas control:
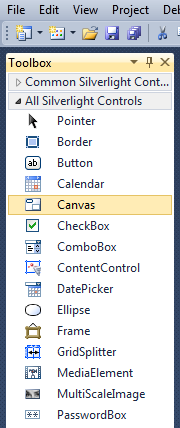
Step 6
Draw a Canvas on the Page then in the XAML Pane above the "</Grid>" then change the "Canvas1" line to the following:
<Canvas Height="65" Width="400" VerticalAlignment="Top" HorizontalAlignment="Left" Name="Toolbar"></Canvas>
See below:
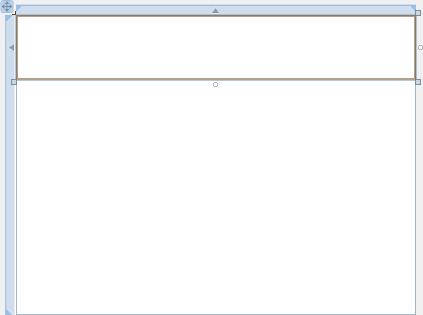
Step 7
Then from the Common Silverlight Controls section in the Toolbox select the Button control:
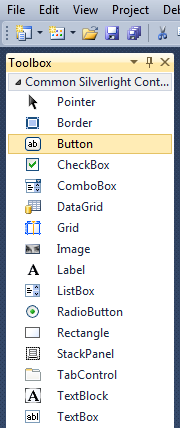
Step 8
Draw Five Buttons on the Canvas (Toolbar) by dragging the Buttons from the Toolbox onto the Canvas or in the XAML Pane inbetween the "<Canvas>" and "</Canvas>" tags type the following:
<Button Canvas.Left="6" Canvas.Top="6" Height="23" Width="75" Name="Button1" Content="Button"/> <Button Canvas.Left="87" Canvas.Top="6" Height="23" Width="75" Name="Button2" Content="Button"/> <Button Canvas.Left="168" Canvas.Top="6" Height="23" Width="75" Name="Button3" Content="Button"/> <Button Canvas.Left="249" Canvas.Top="6" Height="23" Width="75" Name="Button4" Content="Button"/> <Button Canvas.Left="249" Canvas.Top="35" Height="23" Width="75" Name="Button5" Content="Button"/>
See below:
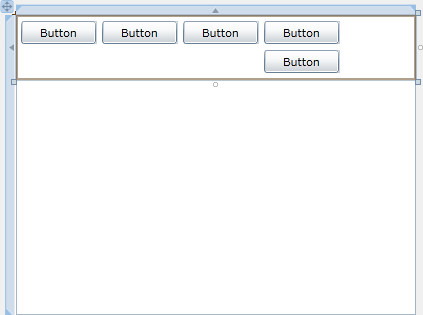
Step 9
Click on the first Button (Button1), then goto the Properties box and change the Name to "New" and the Content property from Button to "New" (both without the quotes), see below:
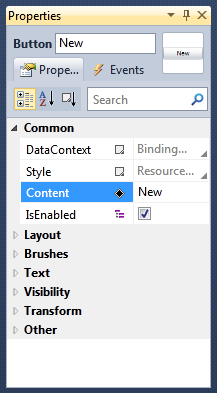
Step 10
Click on the second Button (Button2), then goto the Properties box and change the Name to "Open" and the Content property from Button to "Open...".
Then click on the third Button (Button3) and goto the Properties box and change then Name to "Save" and the Content property from Button to "Save...".
Click on the fourth button (Button4) and then change the Name to "Remove" in the Properties box and the Content property to "Remove".
Then click on the fifth button (Button5) and in the Properties box change the name to "Add" and the Content property to "Add" (all without the quotes),
the page should appear as below:
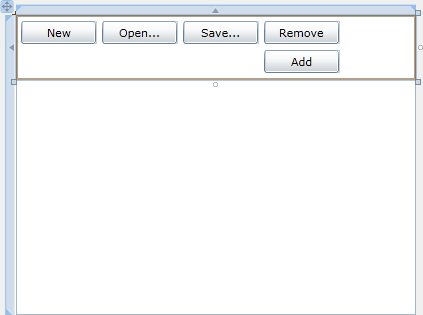
Step 11
Then from the Common Silverlight Controls section in the Toolbox select the TextBox control:
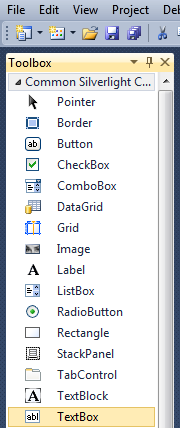
Step 12
Draw a TextBox on the Canvas (Toolbar) with the Buttons, and in the XAML Pane below the "<Button>" tag and above the "</Canvas>" change "TextBox1" to the following:
<TextBox Canvas.Left="6" Canvas.Top="36" Height="23" Width="237" Name="Subject"/>
See below:
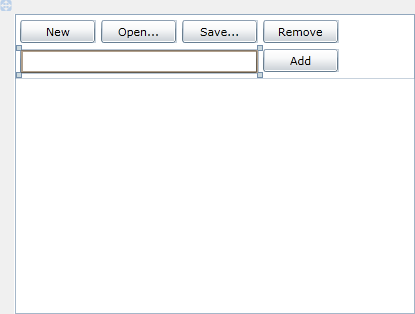
Step 13
Then from the Common Silverlight Controls section in the Toolbox select the ListBox control:
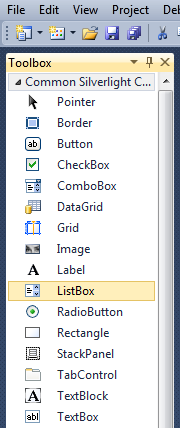
Step 14
Draw a ListBox on the Page, below the Canvas with the Buttons, and in the XAML Pane above the "</Grid>" and below the "</Canvas>" change "ListBox1" to the following:
<ListBox Height="235" Width="400" Margin="0,65,0,0" HorizontalAlignment="Left" VerticalAlignment="Top" Name="Tasks"/>
See below:
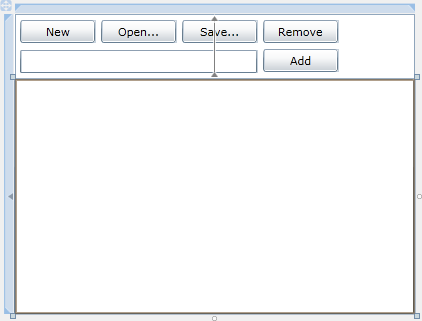
Step 15
Right Click on the Page or the entry for "MainPage.xaml" in Solution Explorer and choose the "View Code" option. In the Code View above the "Partial Public Class MainPage" line type the following:
Imports System.Xml.Linq
See Below:
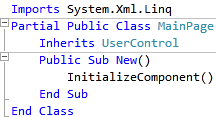
Step 16
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "New" Button Control and type in the New_Click Sub:
If MessageBox.Show("Start a New Task List?", "Task List", _ MessageBoxButton.OKCancel) = MessageBoxResult.OK Then Subject.Text = "" Tasks.Items.Clear() End If
See Below:
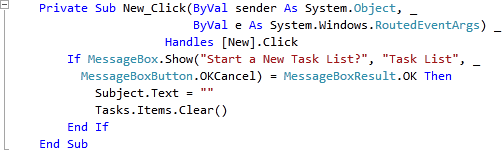
Step 17
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Open..." Button and type in the Open_Click Sub:
Dim OpenDialog As New OpenFileDialog Dim _xml As XElement OpenDialog.Filter = "Task List Files (*.tsk)|*.tsk" If OpenDialog.ShowDialog Then Try If OpenDialog.File.Exists Then _xml = XElement.Parse(OpenDialog.File.OpenText.ReadToEnd) If _xml.Name.LocalName = "tasklist" Then ' Root Tasks.Items.Clear() For Each _Task As XElement In _xml.Descendants("task") Dim _item As New CheckBox _item.IsChecked = _Task.FirstAttribute.Value.ToLower = "checked" _item.Content = _Task.Value Tasks.Items.Add(_item) Next End If End If Catch ex As Exception ' Ignore Errors End Try End If
See Below:
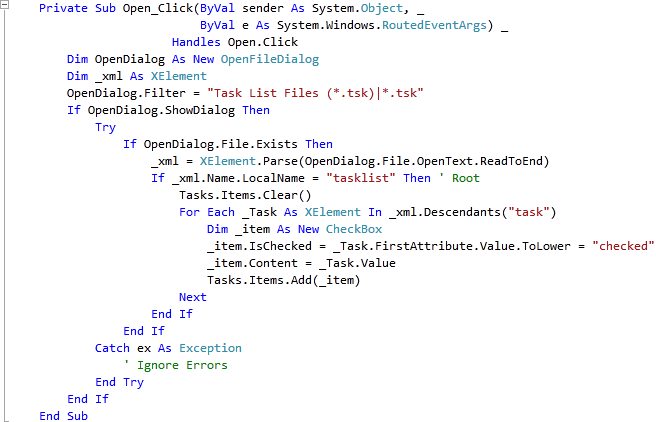
Step 18
Return to the Designer View again, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Save..." Button and type in the Save_Click Sub:
Dim SaveDialog As New SaveFileDialog Dim _doc As XDocument SaveDialog.Filter = "Task List Files (*.tsk)|*.tsk" If SaveDialog.ShowDialog Then Try Dim _items As New XElement("tasklist") For Each _Task As CheckBox In Tasks.Items _items.Add(New XElement("task", _Task.Content, _ New XAttribute("value", IIf(_Task.IsChecked, _ "checked", "unchecked")))) Next _doc = New XDocument(New XDeclaration("1.0", "utf-8", "yes"), _items) Using FileStream As IO.StreamWriter = New IO.StreamWriter(SaveDialog.OpenFile) _doc.Save(FileStream) End Using Catch ex As Exception ' Ignore Errors End Try End If
See Below:
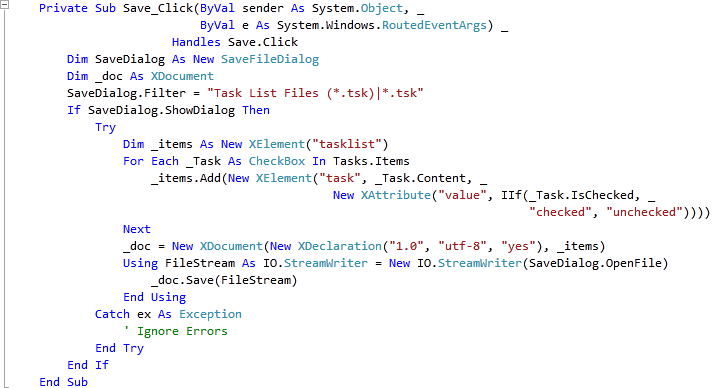
Step 19
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Remove" Button Control and type in the Remove_Click Sub:
If Tasks.SelectedIndex > -1 Then ' Item Selected Tasks.Items.RemoveAt(Tasks.SelectedIndex) ' Remove Selected End If
See Below:
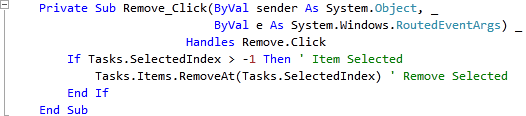
Step 20
Return to the Designer View, by selecting the "MainPage.xaml" Tab, or Right Click on the Page or the Entry for "MainPage.xaml" in Solution Explorer and choose the "View Designer" option.
Double Click on the "Add" Button Control and type in the Add_Click Sub:
If Subject.Text <> "" Then ' Has Subject Dim _item As New CheckBox _item.Content = Subject.Text If Tasks.SelectedIndex > -1 Then ' Item Selected Tasks.Items.Insert(Tasks.SelectedIndex, _item) ' Insert before Selected Else Tasks.Items.Add(_item) ' Add to List End End If End If
See Below:
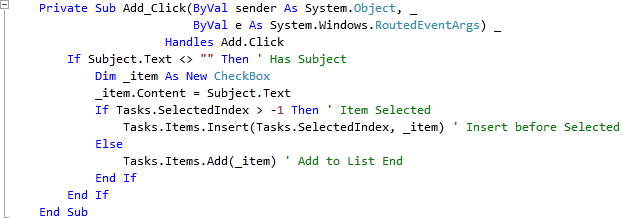
Step 21
Save the Project as you have now finished the Silverlight application. Select Debug then Start Debugging or click on Start Debugging:

After you do, the following will appear in a new Web Browser window:
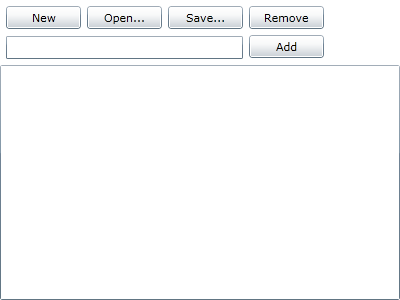
Step 22
Now type into the TextBox the Subject of a Task, then Click on the Add button to add some Tasks to, you can then "Save" this list to "Open" later, see below:
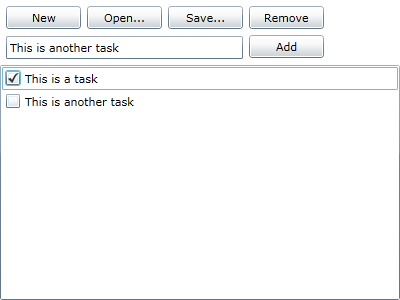
Step 23
Close the Browser window by clicking on the Close Button on
the top right of the Web Browser to Stop the application.
This is a Simple Task List application with the ability to Open and Save Task Lists files as XML including the items checked and the order they appear. Try adding new features like being able to move items Up and Down the list and being able to Edit previously entered Tasks or use this as a basis for more complex XML-based applications!