Web Browsing is another common task, create a simple Web Browser which can Open and Save a Favourite website address using Silverlight on Windows Phone 7.
Printer Friendly Download Tutorial (739KB) Download Source Code (16.6KB)
Step 1
Start Microsoft Visual Studio 2010 Express for Windows Phone, then Select File then New Project... Select "Visual C#" then "Silverlight for Windows Phone" and then "Windows Phone Application" from Templates, select a Location if you wish, then enter a name for the Project and then click OK, see below:
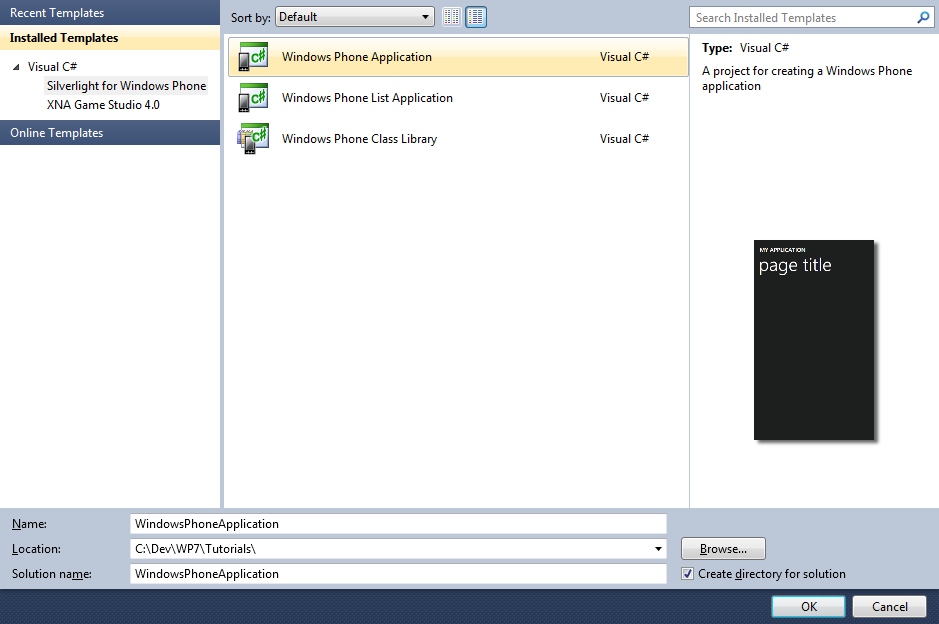
Step 2
A Windows Phone application Page named MainPage.xaml should then appear, see below:
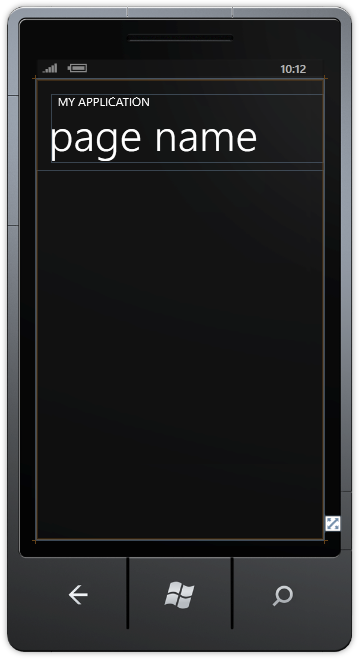
Step 3
Then in the XAML Pane for MainPage.xaml above the <Grid x:Name="LayoutRoot" Background="{StaticResource PhoneBackgroundBrush}"> line type the following XAML:
<phone:PhoneApplicationPage.ApplicationBar>
<shell:ApplicationBar IsVisible="True" IsMenuEnabled="True">
<shell:ApplicationBar.MenuItems>
<shell:ApplicationBarMenuItem Text="open favourite" Click="Open_Click"/>
<shell:ApplicationBarMenuItem Text="save favourite" Click="Save_Click"/>
<shell:ApplicationBarMenuItem Text="delete favourite" Click="Delete_Click"/>
</shell:ApplicationBar.MenuItems>
</shell:ApplicationBar>
</phone:PhoneApplicationPage.ApplicationBar>
See below:
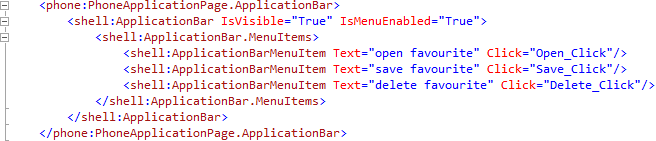
Step 4
While still in the XAML Pane between the <Grid x:Name="ContentGrid" Grid.Row="1"> and </Grid> lines type the following XAML:
<Grid x:Name="ContentMain">
<Grid.RowDefinitions>
<RowDefinition Height="80"/>
<RowDefinition Height="*"/>
</Grid.RowDefinitions>
<Grid x:Name="Toolbar" Grid.Row="0">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="Auto"/>
</Grid.ColumnDefinitions>
<!-- Toolbar -->
</Grid>
<!-- Content -->
</Grid>
XAML:
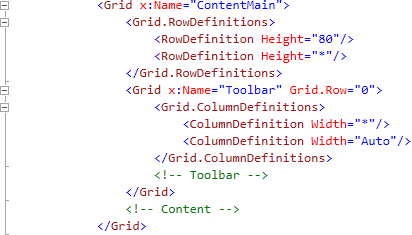
Design:
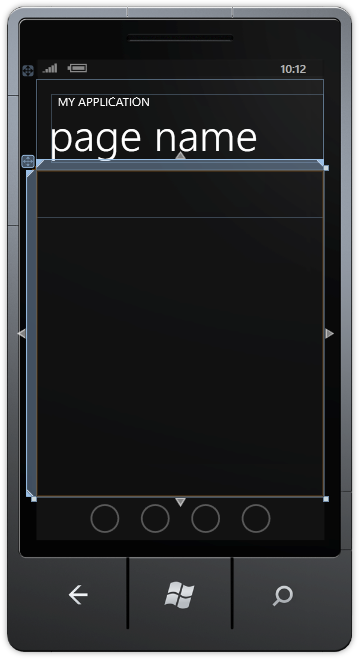
Step 5
Then from the Windows Phone Controls section in the Toolbox select the TextBox control:
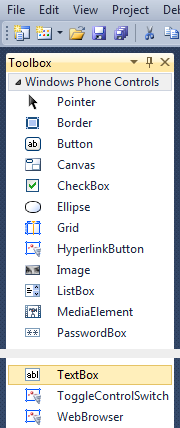
Step 6
Draw a TextBox onto the Toolbar Section (upper smaller section) of the Grid on the Page, below the Page Title, and in the XAML Pane below the <!-- Toolbar --> line, change "TextBox1" to the following:
<TextBox Grid.Column="0" Name="Location">
<TextBox.InputScope>
<InputScope>
<InputScopeName NameValue="Url"/>
</InputScope>
</TextBox.InputScope>
</TextBox>
See below:
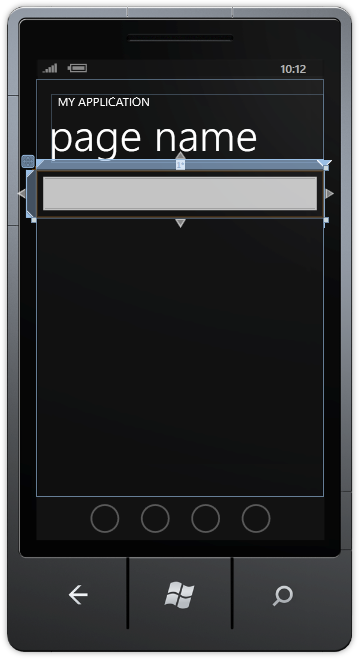
Step 7
Then from the Windows Phone Controls section in the Toolbox select the Button control:
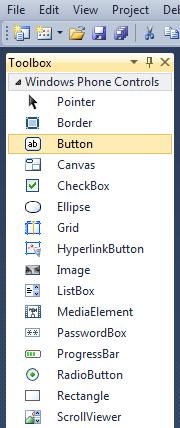
Step 8
Draw a Button onto the Toolbar Section by dragging the Button from the Toolbox onto the Toolbar section of the Grid on the Page, then in the XAML Pane change the "Button1" line to the following:
<Button Grid.Column="1" Content="go" Click="Go_Click"/>
See below:
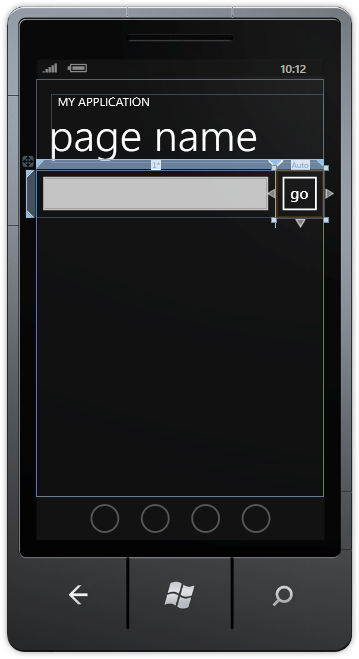
Step 9
Then from the Windows Phone Controls section in the Toolbox select the Web Browser control:
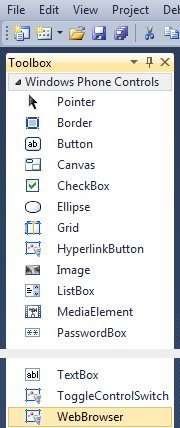
Step 10
Draw a Web Browser onto the Content Section of the Grid on the Page (below the Toolbar section), then in the XAML Pane change the "webBrowser1" line to the following:
<phone:WebBrowser Grid.Row="1" Name="Browser"/>
See below:
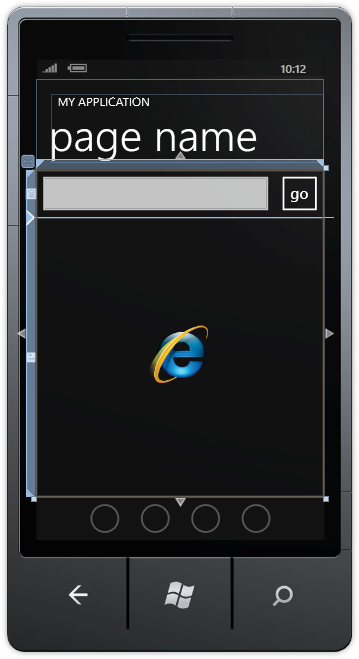
Step 11
Right Click on the Page or the entry for "MainPage.xaml" in Solution Explorer and choose the "View Code" option. In the Code View above "namespace WebBrowser" type the following:
using System.Text.RegularExpressions; using System.IO.IsolatedStorage;
Also in the CodeView above "public MainPage()" type the following declarations:
string filename = "favourite.url"; Regex favourite = new Regex("^URL=(?<URL>.*)$", RegexOptions.Multiline); IsolatedStorageFile storage = IsolatedStorageFile.GetUserStoreForApplication();
See Below:
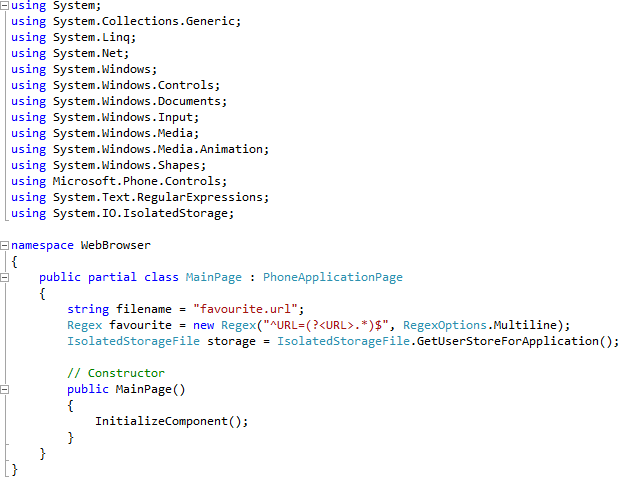
Step 12
While still the Code View for MainPage.xaml.cs above "public MainPage()" type the following Event Handlers:
private void Go_Click(object sender, RoutedEventArgs e) { if (!Location.Text.StartsWith("http://") && !Location.Text.StartsWith("https://")) { Location.Text = ("http://" + Location.Text); } Browser.Navigate(new Uri(Location.Text)); } private void Open_Click(object sender, EventArgs e) { if (storage.FileExists(filename)) { IsolatedStorageFileStream location = new IsolatedStorageFileStream(filename, System.IO.FileMode.Open, storage); System.IO.StreamReader file = new System.IO.StreamReader(location); Location.Text = favourite.Match(file.ReadToEnd()).Groups["URL"].Value; Browser.Navigate(new Uri(Location.Text)); } } private void Save_Click(object sender, EventArgs e) { IsolatedStorageFileStream location = new IsolatedStorageFileStream(filename, System.IO.FileMode.Create, storage); System.IO.StreamWriter file = new System.IO.StreamWriter(location); file.Write("[DEFAULT]\r\n" + "BASEURL=" + Browser.Source.AbsoluteUri + "\r\n" + "[InternetShortcut]\r\n" + "URL=" + Browser.Source.AbsoluteUri); file.Dispose(); location.Dispose(); } private void Delete_Click(object sender, EventArgs e) { if (MessageBox.Show("Delete Favourite?", "Web Browser", MessageBoxButton.OKCancel) == MessageBoxResult.OK) { if (storage.FileExists(filename)) { storage.DeleteFile(filename); } } }
See Below:
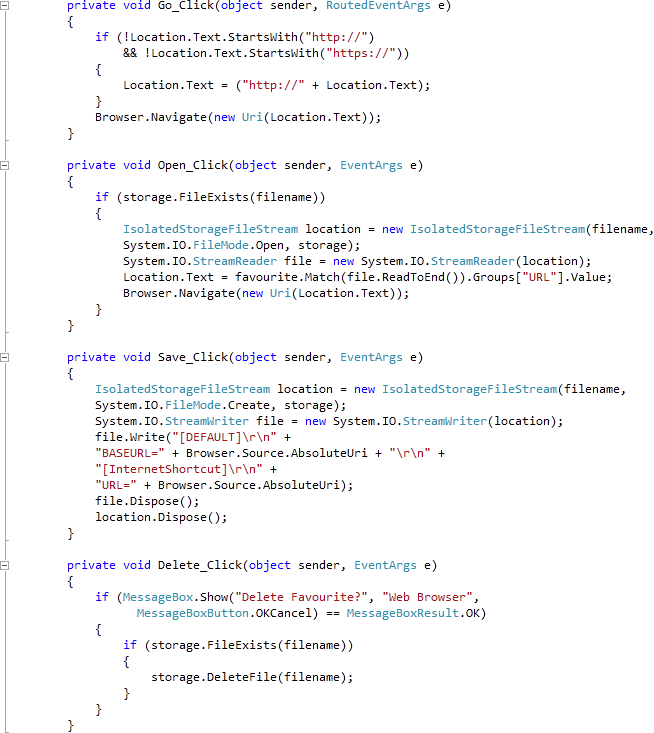
Step 13
Save the Project as you have now finished the Windows Phone Silverlight application. Select the Windows Phone Emulator option then Select Debug then Start Debugging or click on Start Debugging:

After you do, the following will appear in the Windows Phone Emulator after it has been loaded:
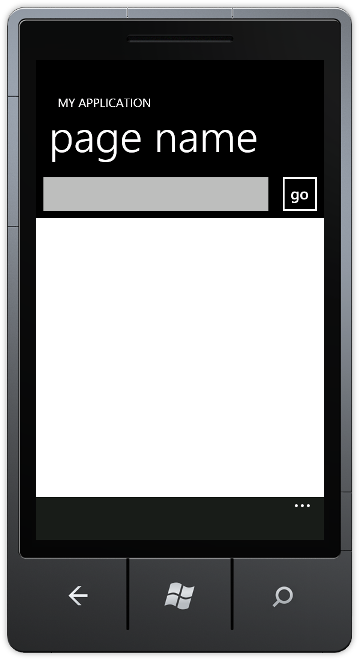
Step 14
Tap the TextBox and then using the SIP or Keyboard enter the address of a Website, then Tap on "go" to visit that website, you can use "save favourite" to allow you to "open favourite" later, see below:
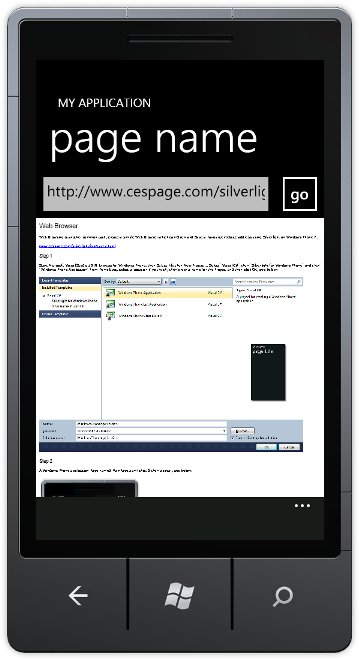
Step 15
You can then Stop the application by selecting the Visual Studio 2010 application window and clicking on the Stop Debugging button:

This is a very simple Web Browser, this example can also "save favourite" and "open favourite" using the standard ".url" format. You can use the Web Browser control to display any HTML content, try adding more features such as the ability to store more than one favourite - make it your own!