Imager is a simple Image Viewing application with procedurally generated Projection animation effects using Silverlight on Windows Phone 7.
Printer Friendly Download Tutorial (424KB) Download Source Code (16.3KB)
Step 1
Start Microsoft Visual Studio 2010 Express for Windows Phone, then Select File then New Project... Select "Visual C#" then "Silverlight for Windows Phone" and then "Windows Phone Application" from Templates, select a Location if you wish, then enter a name for the Project and then click OK, see below:
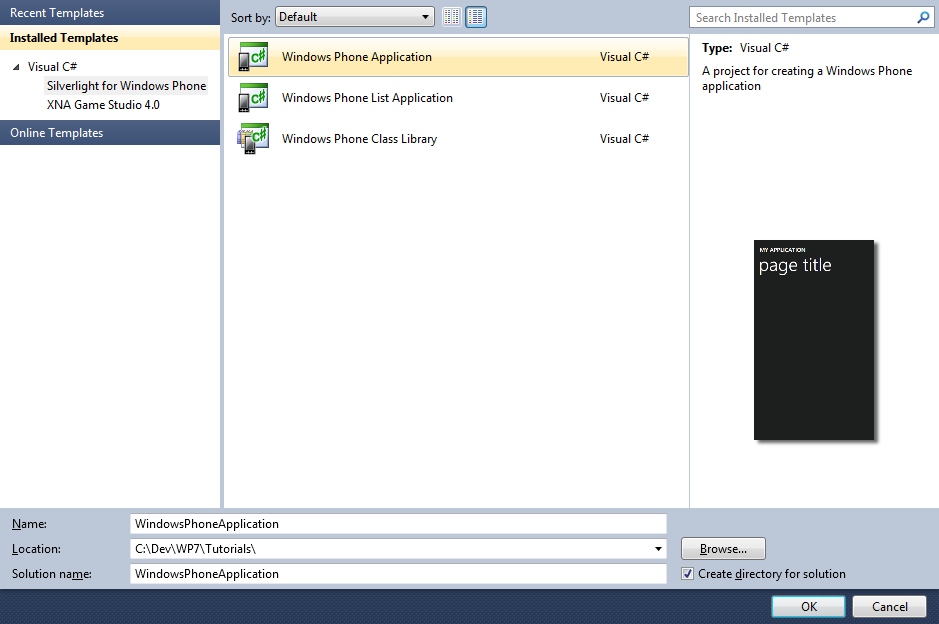
Step 2
A Windows Phone application Page named MainPage.xaml should then appear, see below:
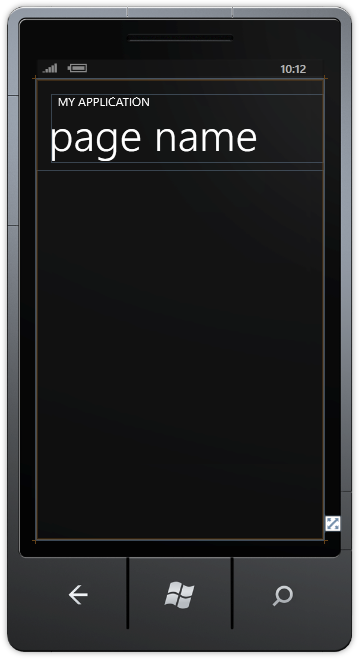
Step 3
In the XAML Pane for MainPage.xaml between the <Grid x:Name="ContentGrid" Grid.Row="1"> and </Grid> lines, enter the following XAML:
<Grid x:Name="ContentMain">
<Grid.RowDefinitions>
<RowDefinition Height="Auto"/>
<RowDefinition Height="*"/>
<RowDefinition Height="Auto"/>
</Grid.RowDefinitions>
<!-- Header -->
<Grid Grid.Row="0" x:Name="Header">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="*"/>
<ColumnDefinition Width="160"/>
</Grid.ColumnDefinitions>
<TextBox Grid.Column="0" Name="Location">
<TextBox.InputScope>
<InputScope>
<InputScopeName NameValue="Url"/>
</InputScope>
</TextBox.InputScope>
</TextBox>
<Button Grid.Column="1" Content="go" Click="Go_Click"/>
</Grid>
<!-- Content -->
<Image Grid.Row="1" Margin="50" Stretch="Uniform" Name="Display">
<Image.Projection>
<PlaneProjection x:Name="Target"/>
</Image.Projection>
</Image>
<!-- Footer -->
<Grid Grid.Row="2" x:Name="Buttons" >
<Grid.ColumnDefinitions>
<ColumnDefinition Width="160"/>
<ColumnDefinition Width="160"/>
<ColumnDefinition Width="160"/>
</Grid.ColumnDefinitions>
<!-- Buttons -->
<Button Grid.Column="0" Content="pitch" Click="Pitch_Click"/>
<Button Grid.Column="1" Content="roll" Click="Roll_Click"/>
<Button Grid.Column="2" Content="yaw" Click="Yaw_Click"/>
</Grid>
</Grid>
XAML:
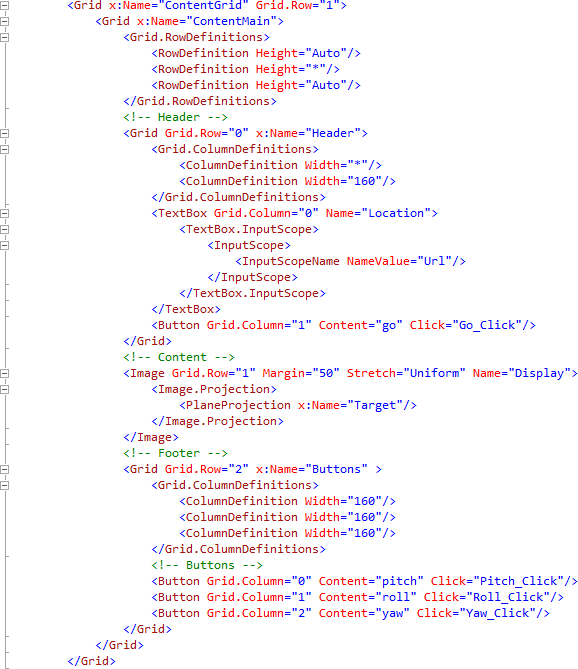
Design:
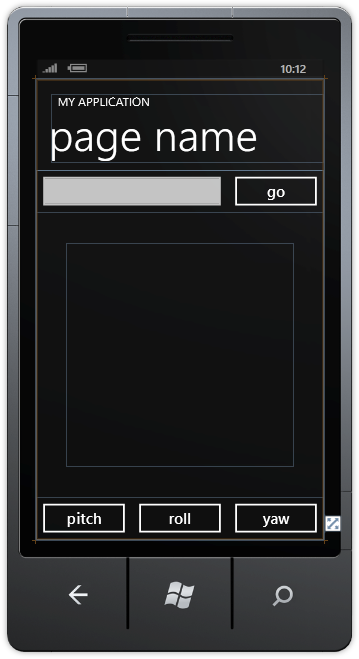
Step 4
Right Click on the Page or the entry for "MainPage.xaml" in Solution Explorer and choose the "View Code" option. In the Code View above "namespace Imager" type the following:
using System.Windows.Media.Imaging;
Also in the CodeView above "public MainPage()" type the following declarations:
private bool Rotating = false; private Storyboard Rotation = new Storyboard();
See Below:
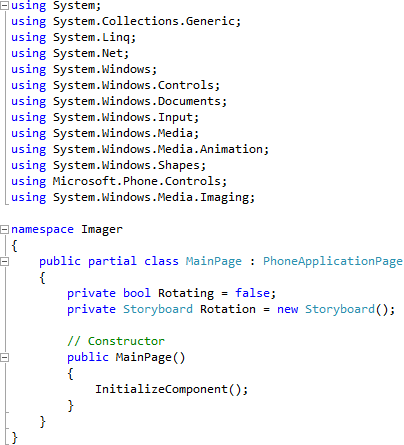
Step 5
While still in the Code View for MainPage.xaml.cs, above the "public MainPage()" method type the following Method:
private void Rotate(object Parameter) { if (Rotating) { Rotation.Stop(); Rotating = false; } else { DoubleAnimation _animation = new DoubleAnimation(); _animation.From = 0.0; _animation.To = 360.0; _animation.Duration = new Duration(TimeSpan.FromSeconds(10)); _animation.RepeatBehavior = RepeatBehavior.Forever; Storyboard.SetTarget(_animation, Target); Storyboard.SetTargetProperty(_animation, new PropertyPath(Parameter)); Rotation.Children.Clear(); Rotation.Children.Add(_animation); Rotation.Begin(); Rotating = true; } }
See Below:
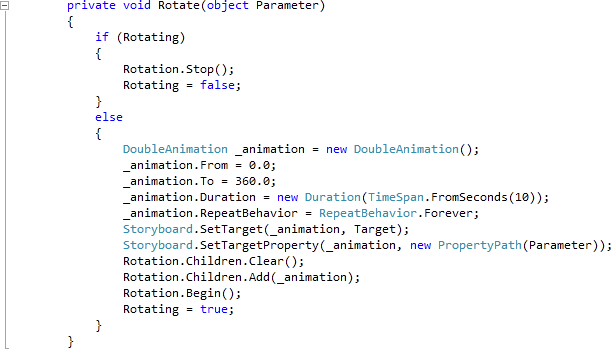
Step 6
While still the Code View for MainPage.xaml.cs, below the "}" of the "public MainPage()" method type the following Event Handlers:
private void Go_Click(object sender, RoutedEventArgs e) { Display.Source = new BitmapImage(new Uri(Location.Text)); } private void Pitch_Click(object sender, RoutedEventArgs e) { Rotate(PlaneProjection.RotationXProperty); } private void Roll_Click(object sender, RoutedEventArgs e) { Rotate(PlaneProjection.RotationZProperty); } private void Yaw_Click(object sender, RoutedEventArgs e) { Rotate(PlaneProjection.RotationYProperty); }
See Below:
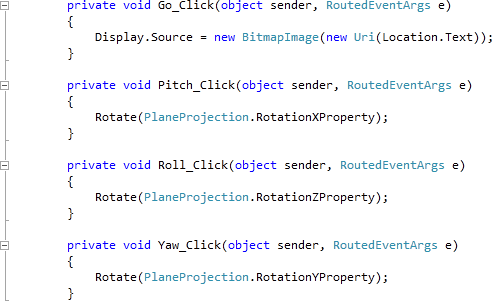
Step 7
Save the Project as you have now finished the Windows Phone Silverlight application. Select the Windows Phone Emulator option then Select Debug then Start Debugging or click on Start Debugging:

After you do, the following will appear in the Windows Phone Emulator after it has been loaded:
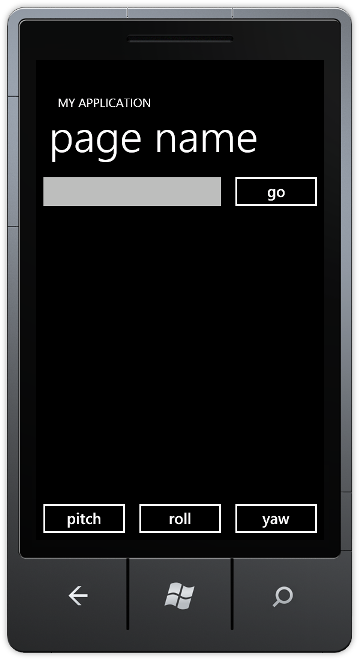
Step 8
Select the TextBox and enter in the web address of an Image such as http://cespage.com/phone.jpg then click on the "go" button, when loaded the image will appear in the Image Control, which you can then Animate with the Pitch, Roll and Yaw Buttons, see below:
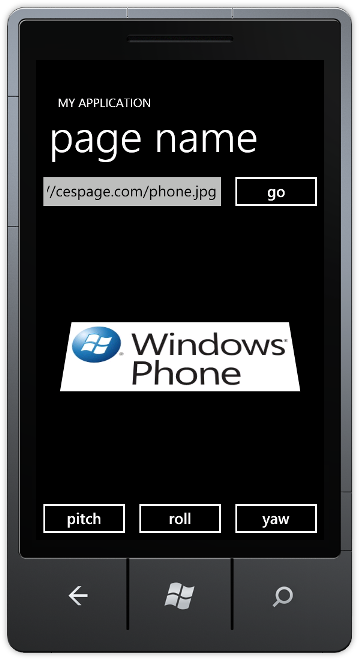
Step 9
You can then Stop the application by selecting the Visual Studio 2010 application window and clicking on the Stop Debugging button:

This was a simple example of animating an Image using Code, it is possible to have multiple animations - if you declare an Animation object for for each type of animation and you can combine animations together, see what you can add and make it your own!